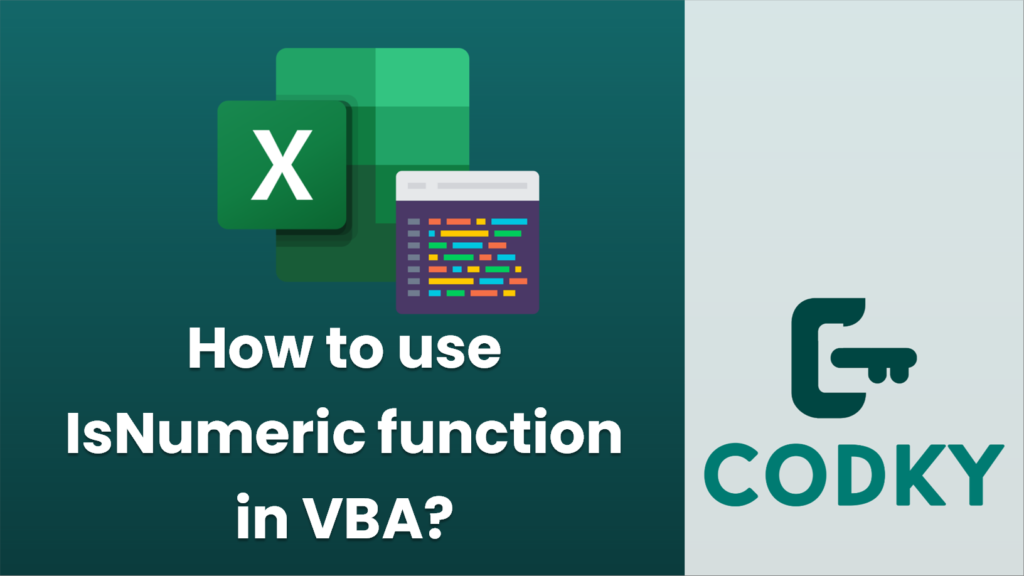
Contents
In VBA (Visual Basic for Applications), the IsNumeric function is used to determine if an expression can be evaluated as a number. This function returns a Boolean value: True if the expression is a number or can be converted to a number, and False otherwise.
Basic Usage
Sub CheckIfNumeric()
Dim testValue As Variant
testValue = "123.45"
If IsNumeric(testValue) Then
MsgBox "The value is numeric."
Else
MsgBox "The value is not numeric."
End If
End Sub
In this example, testValue is assigned a string that represents a decimal number. The IsNumeric function then checks if testValue can be treated as a number. Since “123.45” is a valid numeric string, the function returns True and the message box displays “The value is numeric.”
Here’s another example where testValue is not a number:
Sub CheckIfNumeric()
Dim testValue As Variant
testValue = "Hello World"
If IsNumeric(testValue) Then
MsgBox "The value is numeric."
Else
MsgBox "The value is not numeric."
End If
End Sub
In this case, “Hello World” is not a number, so IsNumeric returns False, and the message box will display “The value is not numeric.”
IsNumeric can be used to check a wide range of expressions, such as variables, literals, or expressions that result from calculations or user inputs. It’s commonly used for input validation or error handling when performing mathematical operations.