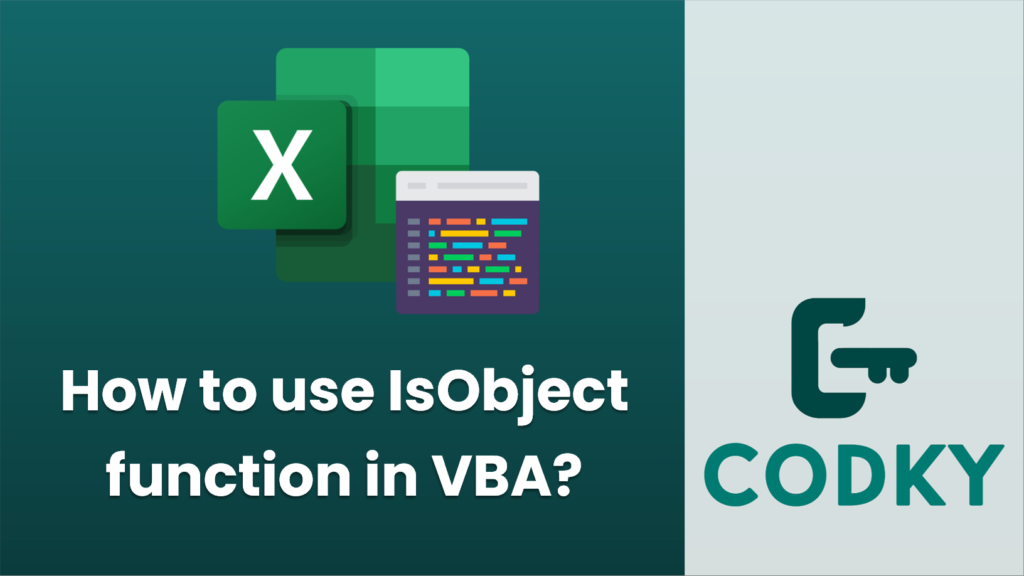
The IsObject function in VBA (Visual Basic for Applications) is used to determine if a variable is an object type. The purpose of the IsObject function is to avoid run-time errors that might occur if you try to perform an object-specific operation on a non-object.
Example
VBA
Sub CheckIfObject()
Dim potentiallyAnObject As Variant
Dim regularVariable As Integer
' Assign a new Collection (which is an object) to the variant
Set potentiallyAnObject = New Collection
' Check if potentiallyAnObject is an Object
If IsObject(potentiallyAnObject) Then
MsgBox "potentiallyAnObject is an object"
Else
MsgBox "potentiallyAnObject is not an object"
End If
' regularVariable is not an object, it's an integer
regularVariable = 10
If Not IsObject(regularVariable) Then
MsgBox "regularVariable is not an object"
End If
End Sub
- We declare two variables, potentiallyAnObject as Variant which can hold both object and non-object types and regularVariable as Integer which can hold numbers.
- We create a New Collection (a collection is a type of object in VBA) and assign it to the potentiallyAnObject variable.
- We then use IsObject to check if potentiallyAnObject is indeed an object. Since we have assigned a Collection object to it, the message box will confirm that it is an object.
- For the regularVariable, which is not an object, IsObject will return False.
Remember that IsObject only checks if the variable is an object; it does not verify the type of the object. If you need to ensure that an object is of a specific type, you would need to use TypeName or TypeOf.