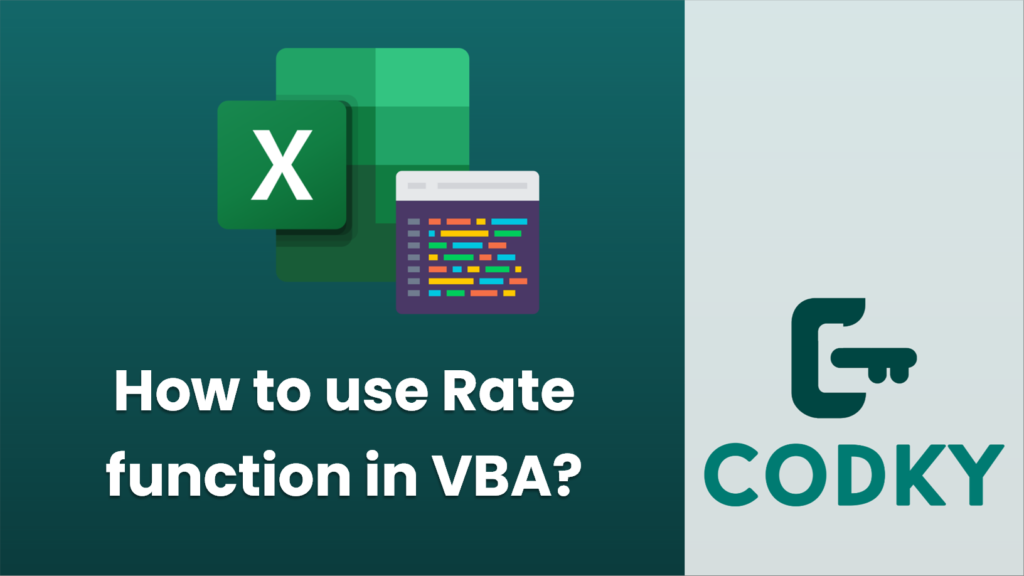
In VBA (Visual Basic for Applications), the
Rate function is used to calculate the interest rate per period of an annuity. This function is part of the financial functions available within Excel, and it can be used in VBA by accessing it through the
WorksheetFunction object.
Here’s the basic syntax for the
Rate function in VBA:
Rate(nper, pmt, pv, [fv], [type], [guess])
Where:
nper is the total number of payment periods in the annuity.
pmt is the payment made each period; it cannot change over the life of the annuity.
pv is the present value, which is the total amount that a series of future payments is worth now.
fv (optional) is the future value, or a cash balance you want to attain after the last payment is made.
type (optional) is the timing of the payment: 0 for end of the period (default), or 1 for beginning of the period.
guess (optional) is your guess for the expected rate. If omitted, it assumes 10% (0.1).
Below is an example of how you could use the
Rate function in VBA to calculate the monthly interest rate for a loan:
Sub CalculateInterestRate()
' Parameters for the Rate function
Dim Periods As Integer: Periods = 60 ' 60 monthly payments
Dim Payment As Double: Payment = -150 ' Monthly payment (negative because it's an outflow)
Dim PresentValue As Double: PresentValue = 8000 ' Amount borrowed
Dim FutureValue As Double: FutureValue = 0 ' Future value after the last payment
Dim PaymentType As Integer: PaymentType = 0 ' Payments are made at the end of the period
Dim Guess As Double: Guess = 0.1 ' Starting guess for the rate (10%)
' Variable to hold the result
Dim MonthlyRate As Double
' Calculate the monthly interest rate
MonthlyRate = Application.WorksheetFunction.Rate(Periods, Payment, PresentValue, FutureValue, PaymentType, Guess)
' Print the result in the Immediate window (Ctrl+G to view)
Debug.Print "The monthly interest rate is: " & MonthlyRate * 100 & "%"
End Sub
In this example,
MonthlyRate will be a decimal representing the monthly interest rate (so you might multiply it by 100 to get a percentage value). Note that the
pmt parameter here has a negative value because it’s considered an outflow of money (a payment).
When using financial functions like
Rate, bear in mind that the results are sensitive to the input values. If the function doesn’t converge to a solution, it will result in an error. You can trap this kind of error with an error-handling routine if needed.
Unlock Your Potential
Help us grow the project