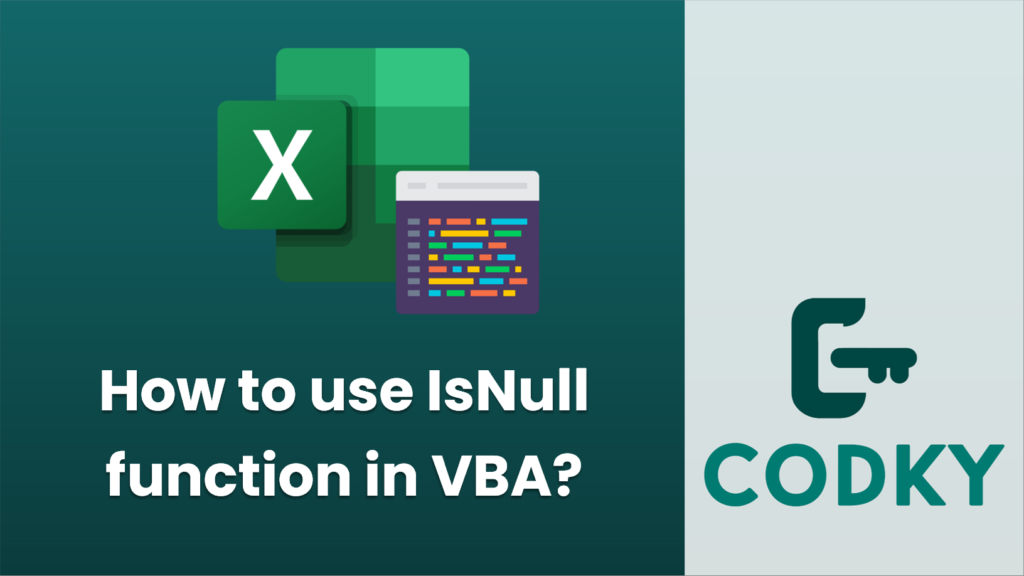
The `IsNull` function in VBA (Visual Basic for Applications) is used to determine whether a variant (a special data type that can contain any type of data) is set to the special `Null` value. The `Null` value indicates that the variant contains no valid data.
Here’s how you can use the `IsNull` function in VBA:
Sub CheckForNull()
Dim varTest As Variant
' Assign the Null value to the variant
varTest = Null
' Check if the variant is Null
If IsNull(varTest) Then
MsgBox "The variable is Null."
Else
MsgBox "The variable is not Null."
End If
End Sub
In this example, the `varTest` variable is explicitly assigned the `Null` value. The `IsNull` function then checks the variable and returns `True` if it is `Null` or `False` if it contains some data.
It’s important to understand that `Null` in databases or VBA is used to represent the absence of a value or an unknown value. This is different from an empty string (`””`) or a `0` (zero) as a numerical value.
`IsNull` can be particularly useful when dealing with databases through VBA, as database fields can often contain `Null` values.
Here’s another example where you might retrieve a value from a database that could potentially be `Null`, and you need to check it before proceeding with the operations:
Sub RetrieveAndCheckData()
' Assume rs is a Recordset object that contains data from a database query
Dim rs As Recordset
Dim value As Variant
'... (Code to set up and execute a database query)
' Retrieve a value from a field in the database record
value = rs.Fields("SomeField").Value
' Check if the value is Null before using it
If IsNull(value) Then
MsgBox "The value from the database is Null."
Else
MsgBox "The value from the database is: " & value
End If
End Sub
Keep in mind that since the `IsNull` function is used to check for `Null` values, it should only be used with variables that can be set to `Null`, which essentially means only variants can hold a `Null` value.