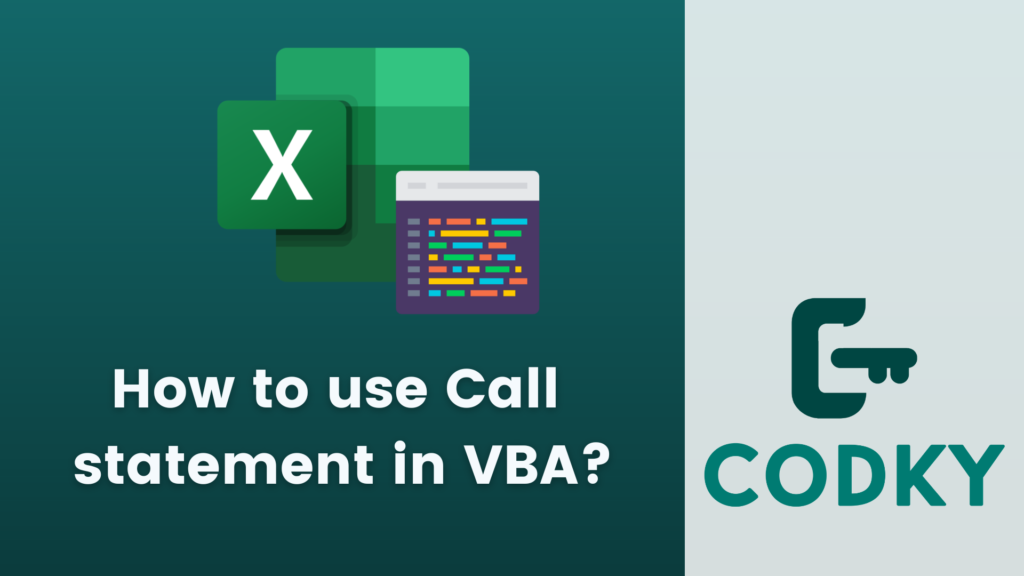
Contents
The Call statement in VBA (Visual Basic for Applications) is used to call a procedure, which can be either a Sub or a Function. However, it’s important to note that in modern VBA, using the Call statement is optional and mostly a matter of coding style. You can call a procedure with or without it. Here’s how to use it:
Using Call with a Sub Procedure:
When you use Call, you must enclose any arguments in parentheses.
Example:
Sub MyProcedure(arg1 As String, arg2 As Integer)
' ... procedure code ...
End Sub
Sub CallExample()
Call MyProcedure("Hello", 123)
End Sub
Calling a Sub Procedure Without Call:
If you don’t use Call, you should not use parentheses around the arguments unless they are needed for a different reason (like a function call).
Example:
Sub CallExample()
MyProcedure "Hello", 123
End Sub
Using Call with a Function Procedure:
Functions return values, so they are usually assigned to a variable or used in an expression. However, you can still use Call if you’re not interested in the return value.
Example:
Function MyFunction(arg1 As String) As Integer
' ... function code ...
MyFunction = 1
End Function
Sub CallFunctionExample()
Dim result As Integer
result = MyFunction("Hello")
' or simply calling without using the return value
Call MyFunction("Hello")
End Sub
Best Practices:
The Call statement is considered redundant in modern VBA coding practices. Most VBA programmers omit it for simplicity and readability.
It’s more common to see sub and function calls without the Call keyword, especially in recent code examples and tutorials.
Remember, whether you use Call or not is mostly a matter of personal or organizational coding style, as it does not affect the functionality of your code.