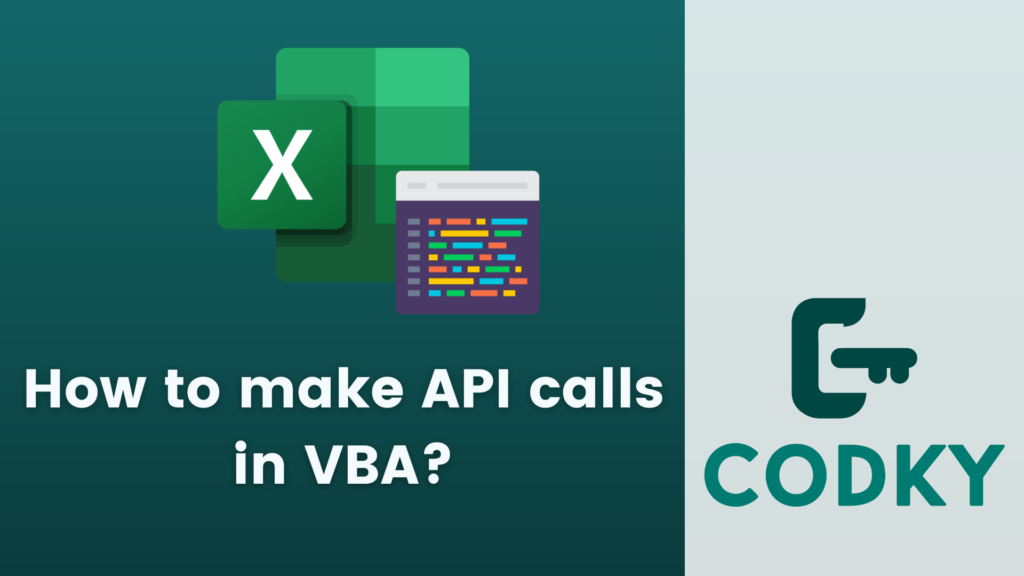
Making API Calls in VBA
Step 1: Enable the MSXML2 Reference
- Open the VBA editor in your Microsoft Office application (like Excel).
- Go to Tools -> References.
- Find and check Microsoft XML, v6.0. If you don’t see it, a lower version like v3.0 can also work, but v6.0 is preferred.
Step 2: Write the VBA Code to Make an API Call
You will typically use the MSXML2.XMLHTTP60 object to make the request. Here’s an example of how to write a simple GET request:VBA
Here’s an example of how to write a simple POST request:
Sub API_GET()
Dim httpRequest As Object
Dim url As String
Dim response As String
Dim jsonResponse As Object
' Create a new XMLHTTP object
Set httpRequest = CreateObject("MSXML2.XMLHTTP.6.0")
' Specify the URL of the API
url = "https://api.example.com/getdata" ' Replace with your API URL
' Open the request (GET method)
httpRequest.Open "GET", url, False
' (Optional) If API requires authentication
' httpRequest.setRequestHeader "Authorization", "Bearer YOUR_ACCESS_TOKEN"
' Send the request to the server
httpRequest.Send
' Get the response data
response = httpRequest.responseText
' Parse JSON response
Set jsonResponse = JsonConverter.ParseJson(response)
' Process the JSON response
' Example: Display a value from the JSON response
' This depends on the structure of your JSON data
MsgBox "Received: " & jsonResponse("someKey")
' Clean up
Set httpRequest = Nothing
Set jsonResponse = Nothing
End Sub
VBA
Sub API_POST()
Dim httpRequest As Object
Dim url As String
Dim postData As String
Dim response As String
' Create a new XMLHTTP object
Set httpRequest = CreateObject("MSXML2.XMLHTTP.6.0")
' Specify the URL of the API
url = "https://api.example.com/postdata" ' Replace with your API URL
' JSON data to send
postData = "{""name"":""John Doe"",""age"":30,""city"":""New York""}"
' Open the request (POST method)
httpRequest.Open "POST", url, False
' Set the Content-Type header to 'application/json'
httpRequest.setRequestHeader "Content-Type", "application/json"
' (Optional) If API requires authentication
' httpRequest.setRequestHeader "Authorization", "Bearer YOUR_ACCESS_TOKEN"
' Send the JSON data
httpRequest.Send postData
' Get the response data
response = httpRequest.responseText
' Process the response
MsgBox response
' Clean up
Set httpRequest = Nothing
End Sub
Step 3: Error Handling
Consider adding error handling to your code to manage issues like network errors, API errors, or invalid responses.Handling JSON in VBA
Step 1: Include VBA-JSON Library
- Download VBA-JSON from GitHub.
- Import JsonConverter.bas into your VBA project.
- Add a reference to Microsoft Scripting Runtime in Tools -> References.
Step 2: Parse JSON String
Convert JSON strings into VBA objects using JsonConverter:VBA
Sub ParseJSONExample()
Dim jsonString As String
Dim jsonObject As Object
jsonString = "{""name"":""John Doe"",""age"":30,""city"":""New York""}"
Set jsonObject = JsonConverter.ParseJson(jsonString)
MsgBox "Name: " & jsonObject("name") & ", Age: " & jsonObject("age")
End Sub
Step 3: Convert VBA Objects to JSON
Convert VBA objects (Dictionaries, Collections) to JSON strings:VBA
Sub ConvertToJSONExample()
Dim jsonObject As Object
Dim jsonString As String
Set jsonObject = CreateObject("Scripting.Dictionary")
jsonObject.Add "name", "Jane Doe"
jsonObject.Add "age", 29
jsonObject.Add "city", "Los Angeles"
jsonString = JsonConverter.ConvertToJson(jsonObject)
MsgBox jsonString
End Sub
Step 4: Handling Complex JSON
VBA-JSON can handle arrays and nested objects, using Collections for arrays and Dictionaries for objects.Notes:
- Replace “https://api.example.com/data” with the actual URL of the API you are calling.
- For APIs requiring authentication, make sure to include the necessary headers or tokens as required by the API.
- Be aware of cross-domain issues if you’re calling APIs from VBA in a networked or secure environment.
- Some APIs return data in JSON format, which VBA does not natively parse. You may need to use a JSON parser or write custom code to handle JSON responses.