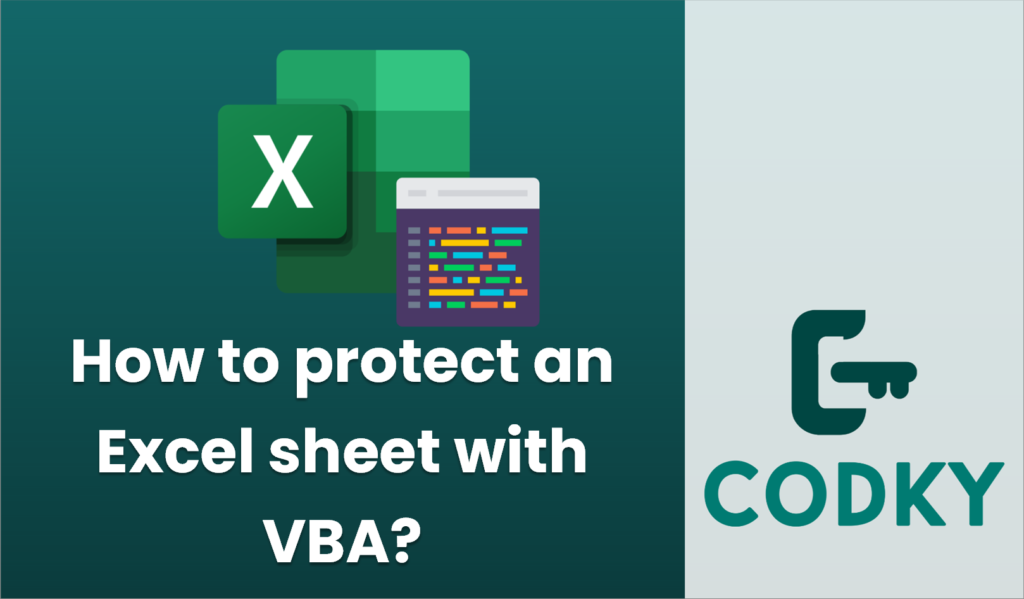
Protecting an Excel sheet using VBA involves using a few lines of code to password-protect the sheet, which limits what users can do within it. Here’s a step-by-step guide to achieve this:
Sub ProtectSheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1") ' Replace "Sheet1" with the name of your sheet
ws.Protect Password:="yourpassword", AllowSorting:=True, AllowFiltering:=True
End Sub
Sub UnprotectSheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1") ' Replace "Sheet1" with the name of your sheet
ws.Unprotect Password:="yourpassword"
End Sub
- Open VBA Editor:
- Press `ALT` + `F11` to open the Visual Basic for Applications (VBA) editor.
- Insert a Module:
- In the VBA editor, go to `Insert` > `Module` to create a new module where you can write your VBA code.
- Write the VBA Code:
- In the module window, you can write a macro that protects the desired worksheet. Here’s an example code snippet:
- Replace `”Sheet1″` with the actual name of the sheet you want to protect.
- Replace `”yourpassword”` with the password you’d like to use.
- The `AllowSorting` and `AllowFiltering` parameters are optional and can be modified based on the actions you want to permit. Other options like `AllowInsertingRows`, `AllowDeletingRows`, etc., can be included as needed.
- Run the Macro:
- Close the VBA editor to return to Excel.
- Press `ALT` + `F8`, select `ProtectSheet`, and click `Run`.
- Test the Sheet Protection:
- Try to modify the sheet to ensure the protection is active. You should be prompted to enter a password for unauthorized actions like editing protected cells.
- Unprotecting the Sheet (Optional):
- If you need to unprotect the sheet, you can use another macro:
- Remember to replace the names and passwords as per your requirement.
By following these steps, you should be able to protect your Excel sheet with a password using VBA. Make sure you keep the password secure, as forgetting it may make it difficult to access the sheet content.