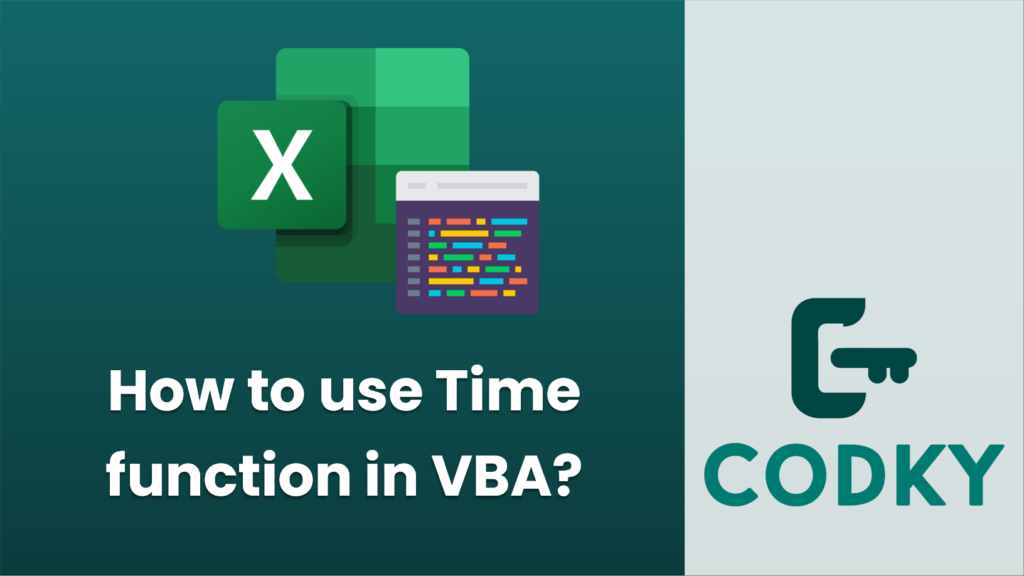
In VBA (Visual Basic for Applications), the `Time` function is used to get the current system time. You don’t need to pass any arguments to the function; it will return the current time as a `Variant` of subtype `Date`.
To use the `Time` function, you can simply call it in your code and assign the value it returns to a variable. Here is an example:
Sub DisplayCurrentTime()
Dim currentTime As Variant
currentTime = Time
MsgBox "The current time is: " & Format(currentTime, "hh:mm:ss AM/PM")
End Sub
The `Format` function is used to format the time in a more human-readable form, with hours, minutes, seconds, and AM/PM indicator.
Remember that the time returned by the `Time` function will be in the time format of your system settings. If you need the time in a specific format, use the `Format` function as shown above, or use the `Hour`, `Minute`, and `Second` functions to extract specific components of the time and then concatenate them as you wish.
The `Time` function is often used together with the `Date` function, which returns the current system date, to form a `DateTime`:
Sub DisplayCurrentDateTime()
Dim currentDateTime As Variant
currentDateTime = Now ' Alternatively, you can use Date + Time
MsgBox "The current date and time is: " & currentDateTime
End Sub
In this example, the `Now` function is used, which is a combination of both `Date` and `Time` functions. Using `Now` is the same as adding the results of the `Date` and `Time` together, and it gives you the current date and time.