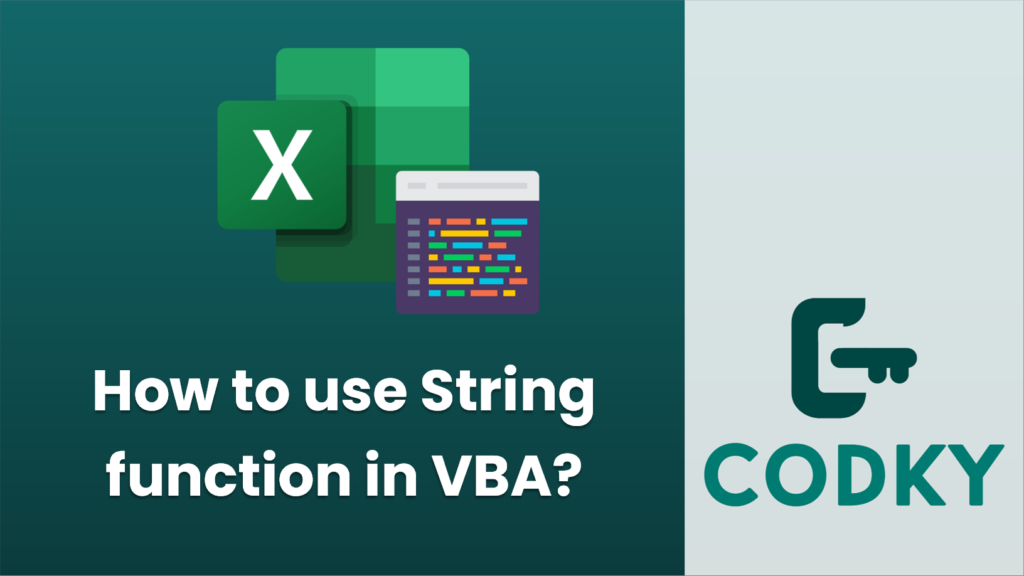
Contents
In VBA (Visual Basic for Applications), the String function is used to create a string that consists of repeated characters. The syntax for the String function is as follows:
Basic syntax
VBA
String(Number, Character)
- Number is the number of times the Character will be repeated.
- Character can be a numeric code representing the character (using the ASCII code) or the character itself in quotes.
String function
VBA
Sub UseStringFunction()
Dim repeatedStr As String
' Repeat the letter "A" 10 times.
repeatedStr = String(10, "A")
MsgBox repeatedStr ' Output will be: AAAAAAAAAA
' Repeat the character with ASCII code 42 (*) 5 times.
repeatedStr = String(5, 42)
MsgBox repeatedStr ' Output will be: <strong></strong>*
End Sub
The String function is particularly useful when you need to create padding for formatting purposes, such as creating fixed-width strings, or when you want to initialize a string variable with a specific character repeated several times.
String function with variables:
VBA
Sub UseStringFunctionWithVariables()
Dim characterToRepeat As String
Dim numberOfRepetitions As Integer
Dim resultString As String
characterToRepeat = "%" ' Character to repeat
numberOfRepetitions = 20 ' Number of times to repeat
' Create string using the String function
resultString = String(numberOfRepetitions, characterToRepeat)
' Display the result
MsgBox "The result string is: " & resultString
End Sub
Replace % with the character you wish to repeat, and 20 with the desired number of repetitions to customize the example.