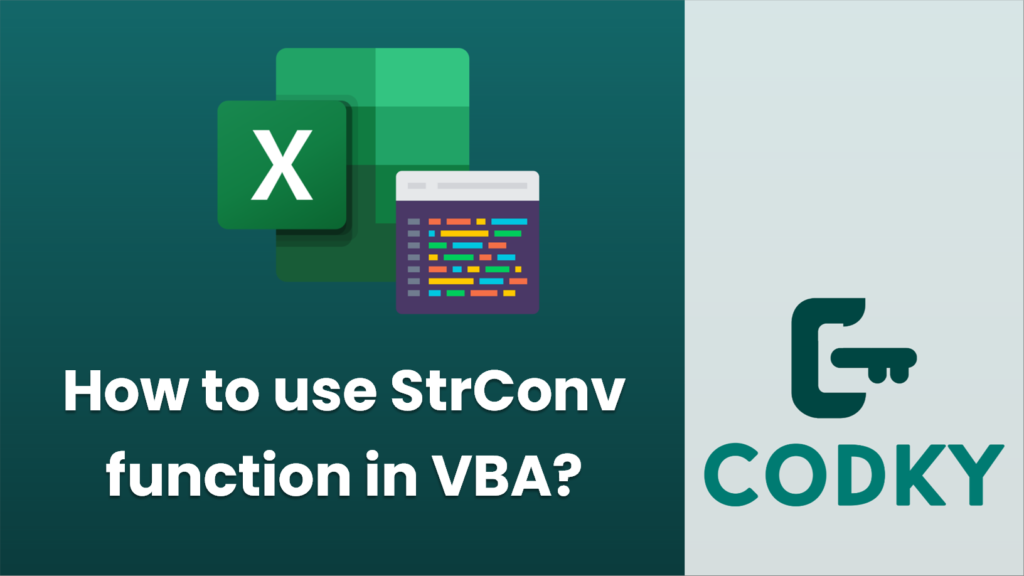
Contents
The StrConv function in VBA is used to convert a text string into a specified format. This function is very handy when you need to change the case of a string (to uppercase or lowercase for example), or to perform conversions from one locale or system to another.
Basic syntax
StrConv(string, conversion, [LCID])
- string is the text string you want to convert.
- conversion is the type of conversion you want to perform on the string. It can be one of the VbStrConv enumeration values.
- LCID is optional. It’s a LocaleID that specifies the locale to use for the conversion. If omitted, the LCID is the system locale.
Here are some of the VbStrConv enumeration values that you can use for the conversion argument:
- vbUpperCase: Converts the string to uppercase.
- vbLowerCase: Converts the string to lowercase.
- vbProperCase: Converts the first letter of each word in the string to uppercase.
- vbWide: Converts narrow (single-byte) characters in a string to wide (double-byte) characters.
- vbNarrow: Converts wide (double-byte) characters in a string to narrow (single-byte) characters.
- vbKatakana: Converts Hiragana characters in a string to Katakana characters.
- vbHiragana: Converts Katakana characters in a string to Hiragana characters.
- vbUnicode: Converts the string to Unicode.
- vbFromUnicode: Converts the string from Unicode to the default code page of the system.
Example
Sub ConvertString()
Dim originalString As String
Dim convertedString As String
originalString = "Hello World!"
' Convert to uppercase
convertedString = StrConv(originalString, vbUpperCase)
Debug.Print convertedString ' Output: "HELLO WORLD!"
' Convert to lowercase
convertedString = StrConv(originalString, vbLowerCase)
Debug.Print convertedString ' Output: "hello world!"
' Convert to Proper case (Title case)
convertedString = StrConv(originalString, vbProperCase)
Debug.Print convertedString ' Output: "Hello World!"
End Sub
To use this function, you would simply copy this subroutine into a module within the VBA editor of your Excel or other Office application, and run it to see the results in the Immediate Window.
Remember to test and modify the code as needed, especially when using the LCID argument, to align with the specific locale-related conversions for your data and system environment.