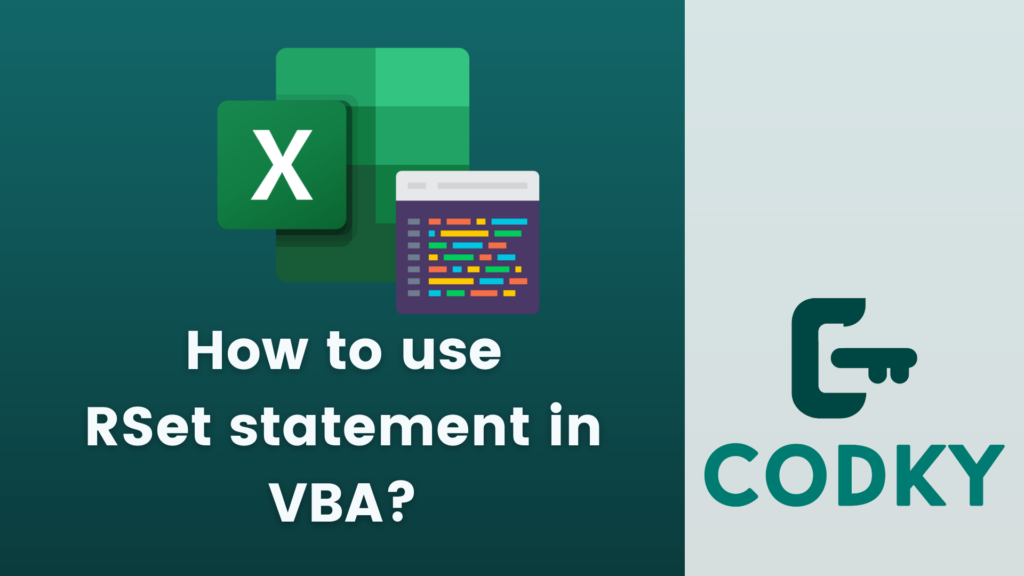
Basic Syntax
VBA
RSet stringVar = stringExpression
- stringVar is the name of the string variable in which you want to right-align another string.
- stringExpression is the string or string expression that you want to right-align within stringVar.
Example Usage
VBA
In this example, myStr is a fixed-length string variable with a length of 10 characters. The RSet statement right-aligns the string “Hello” within myStr, resulting in five spaces being added to the left of “Hello”.
Dim myStr As String * 10 ' Declare a fixed-length string
RSet myStr = "Hello" ' Right-align "Hello" in myStr
MsgBox "|" & myStr & "|" ' Displays "| Hello|"
Error Handling
- If stringExpression is longer than the length of stringVar, a run-time error occurs.
- If stringVar is not a fixed-length string, a run-time error occurs.
Use with Variable-Length Strings
While RSet is intended for use with fixed-length strings, you can simulate right-alignment with variable-length strings by using string functions like Space and Len. For example:VBA
Dim varLengthStr As String
varLengthStr = "Hello"
varLengthStr = Space(10 - Len(varLengthStr)) & varLengthStr
MsgBox "|" & varLengthStr & "|" ' Displays "| Hello|"