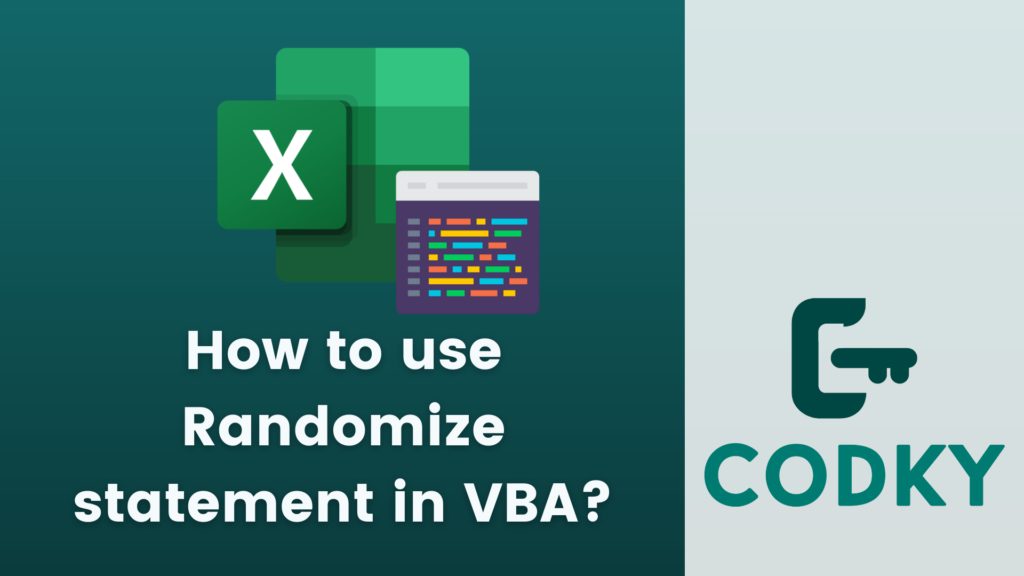
Contents
In VBA (Visual Basic for Applications), the Randomize statement is used to initialize the random number generator, which affects the sequence of numbers returned by the Rnd function. The Rnd function is used to generate a random number between 0 and 1.
Using the Randomize statement ensures that you get a different sequence of random numbers each time your code is run. If you don’t use Randomize, the same sequence of random numbers can be generated each time, depending on the start value (seed) of the random number generator.
How to Use the Randomize Statement
Call Randomize at the Start of Procedure/Module
It’s common to place the Randomize statement at the beginning of the procedure or module where you intend to generate random numbers. This initializes the random number generator with a seed based on the system timer.
Sub GenerateRandomNumbers()
Randomize ' Initialize the random number generator
Dim randomNumber As Double
randomNumber = Rnd() ' Generate a random number between 0 and 1
' Do something with randomNumber
End Sub
Using a Specific Seed
Optionally, you can provide a specific seed value to Randomize if you want to reproduce a predictable sequence of random numbers (useful for testing purposes).
Sub GeneratePredictableRandomNumbers()
Randomize 5 ' Initialize the random number generator with a specific seed
Dim randomNumber As Double
randomNumber = Rnd() ' Generate a random number
' Do something with randomNumber
End Sub
Generating Random Numbers within a Range
While Rnd generates numbers between 0 and 1, you can scale and shift this to any desired range. For example, to generate a random integer between 1 and 10:
Sub GenerateRandomIntegers()
Randomize
Dim randomInteger As Integer
randomInteger = Int((10 * Rnd()) + 1) ' Random number between 1 and 10
' Do something with randomInteger
End Sub
Points to Remember
- Frequency of Randomize Call: Typically, you only need to call Randomize once at the start of the procedure or before a series of Rnd calls. Calling Randomize before each Rnd call can lead to less randomness since the seed value doesn’t change much over short periods.
- Predictability with Same Seed: If you use the same seed value with Randomize, you will get the same sequence of random numbers with each run, which can be useful for testing and debugging.
- Avoiding Randomize in Tight Loops: In loops where performance is critical, you may want to avoid using Randomize inside the loop to prevent potential performance issues.
Using Randomize effectively allows you to incorporate randomness into your VBA applications, making them more dynamic and capable of a variety of tasks like simulations, games, random sampling, and more.