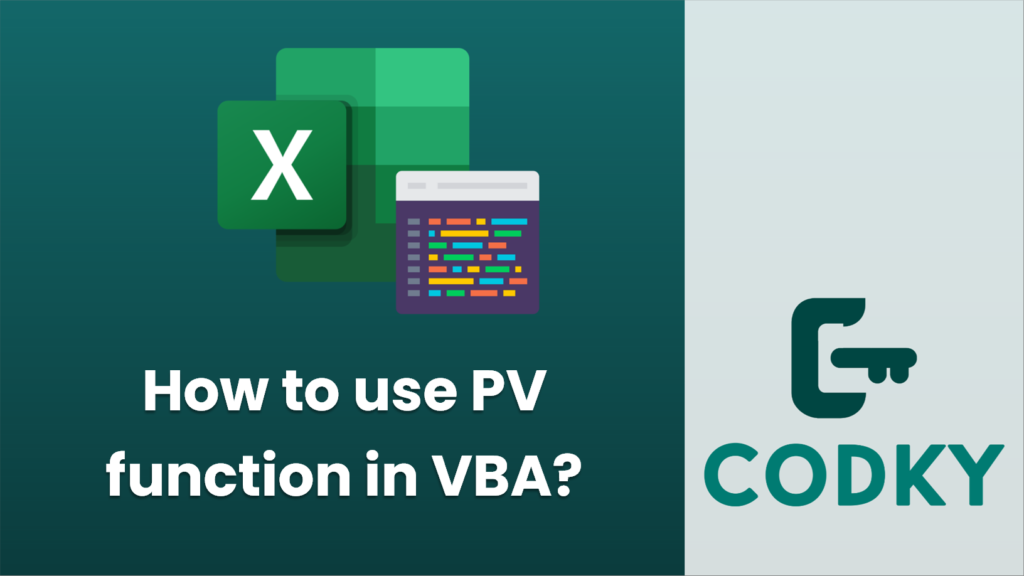
The PV (Present Value) function in Excel VBA is used to calculate the present value of an investment based on a constant interest rate and a series of future payments (negative values) and income (positive values). The syntax of the PV function in VBA is:
PV(rate, nper, pmt, [fv], [type])
Where:
- `rate` is the interest rate per period. For example, if you have an annual interest rate of 6% and you are calculating the present value of monthly payments, you would use 0.06/12 for the rate.
- `nper` is the total number of payment periods in the investment.
- `pmt` is the payment made each period; it cannot change over the life of the investment.
- `fv` (optional) is the future value, or the cash balance you want to attain after the last payment is made. If omitted, it is assumed to be 0; that is, the future value of a loan, for example, is 0.
- `type` (optional) is a number 0 or 1 and indicates when payments are due. 0 indicates payments are due at the end of the period; 1 indicates payments are due at the beginning of the period.
Here’s an example of how to use the PV function in Excel VBA:
Sub CalculatePresentValue()
' Declare variables
Dim interestRate As Double
Dim numberOfPeriods As Integer
Dim paymentPerPeriod As Double
Dim futureValue As Double
Dim paymentType As Integer
Dim presentValue As Double
' Assign values to variables
interestRate = 0.06 / 12 ' 6% annual interest rate, calculated monthly
numberOfPeriods = 60 ' Total 60 months
paymentPerPeriod = -1000 ' Monthly payment
futureValue = 0 ' We want to find out the present value, so future value is 0
paymentType = 0 ' Payments at the end of the period
' Calculate present value using the PV function
presentValue = PV(rate:=interestRate, nper:=numberOfPeriods, pmt:=paymentPerPeriod, fv:=futureValue, type:=paymentType)
' Print the result in Immediate Window (Ctrl + G to view)
Debug.Print "The present value of the investment is: "; presentValue
End Sub
This code snippet will calculate the present value of a series of 60 monthly payments of $1,000 at an annual interest rate of 6%, and it will print the result to the Immediate Window within the VBA editor. Note that the payment is expressed as a negative number because it is an outflow of cash from the perspective of the investor.
Remember, you would need to adjust the `interestRate`, `numberOfPeriods`, `paymentPerPeriod`, `futureValue`, and `paymentType` variables to match your specific investment conditions.