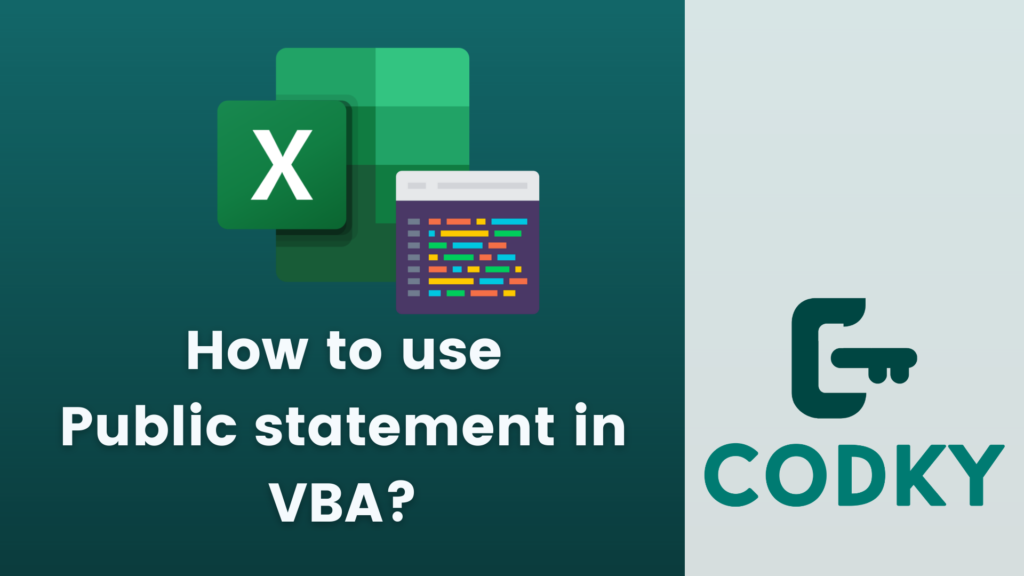
Declaring Public Variables
Module-Level Scope
You typically declare public variables at the top of a module (outside of any procedures or functions) to make them accessible throughout the module and other modules in the project.VBA
Public myVariable As Integer
Accessing Public Variables
Once declared, these variables can be accessed or modified from any procedure within any module in the VBA project.VBA
Sub ModifyVariable()
myVariable = 10
End Sub
Declaring Public Procedures
Public Sub Procedures
You can declare a Sub procedure as public. This makes it callable from any module.VBA
Public Sub MyProcedure()
' Code here
End Sub
Public Function Procedures
Similarly, you can declare a function as public.VBA
Public Function MyFunction() As Integer
' Code here
MyFunction = 1
End Function
Best Practices
- Global Variables: Use public variables sparingly. Overuse of global variables (variables that are accessible throughout the entire application) can make your code harder to debug and maintain.
- Modular Design: It’s often better to pass variables as parameters to procedures rather than relying on public variables, as this makes your code more modular and easier to understand.