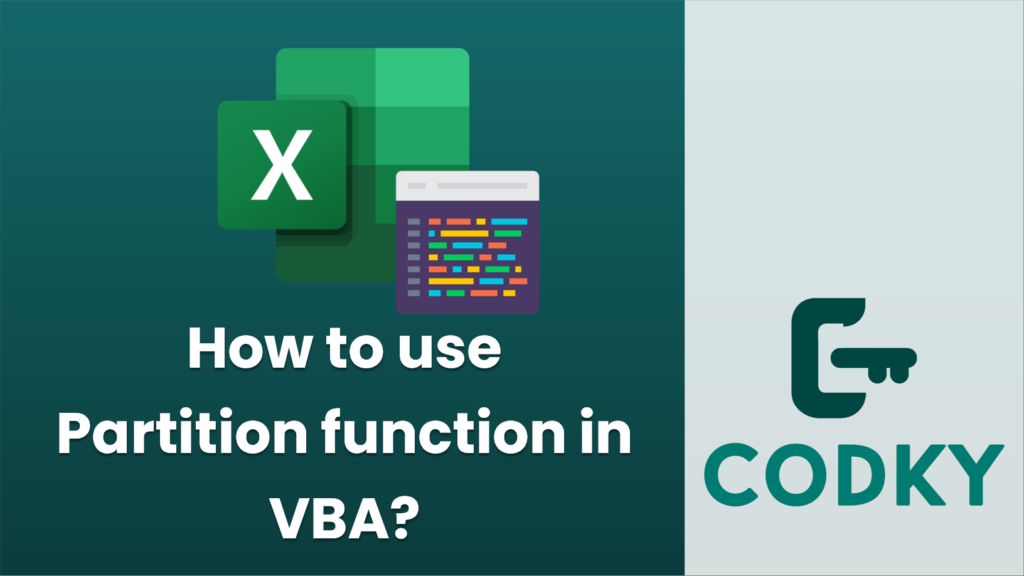
In Visual Basic for Applications (VBA), there is no built-in function called “Partition”. You might be referring to a function that partitions numbers into ranges or segments, but this is not a function that’s provided natively within VBA.
However, you can create your own custom function to achieve a similar result. Here is an example of how you might write a Partition function in VBA, which segments a number into different ranges:
Function Partition(Value As Double, ByVal MinValue As Double, ByVal MaxValue As Double, ByVal Interval As Double) As String
' Check if the value is less than the minimum value
If Value < MinValue Then
Partition = "<" & MinValue
' Check if the value is greater than or equal to the maximum value
ElseIf Value >= MaxValue Then
Partition = ">=" & MaxValue
Else
Dim LowerBound As Double
LowerBound = MinValue
' Loop to determine the correct range for the value
Do While LowerBound + Interval <= Value
LowerBound = LowerBound + Interval
Loop
Partition = "[" & LowerBound & " " & LowerBound + Interval & ")"
End If
End Function
You can use this function in your VBA code to get a string representation of the range in which a given number falls. For example:
Sub TestPartition()
Dim testValue As Double
testValue = 45.5
Debug.Print Partition(testValue, 0, 100, 10) ' Outputs: [40 50)
End Sub
In this example,
Partition is a custom function that takes a number and returns a string indicating the interval it falls into, based on the specified minimum value, maximum value, and interval range. If the value is below the minimum or greater than or equal to the maximum, it prefixes the range with "<" or ">=" respectively.
Remember, this is just a basic example, and you may want to expand or customize this function according to your specific needs.
Unlock Your Potential
Help us grow the project