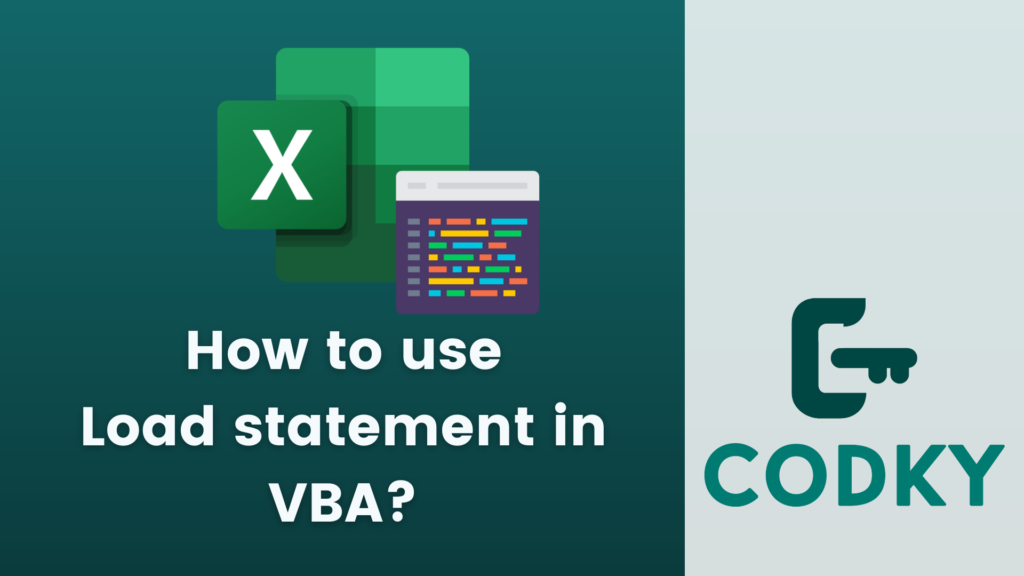
Contents
In VBA (Visual Basic for Applications), the Load statement is used to load a form into memory without displaying it. This can be useful when you want to prepare a form (like initializing certain controls or variables) before showing it to the user.
Basic Usage
Load FormName
FormName: This is the name of the form that you want to load.
Example
Suppose you have a form named UserForm1. Here’s how you might use the Load statement:
Sub LoadMyForm()
Load UserForm1
' Perform any initialization here
' ...
UserForm1.Show
End Sub
In this example, UserForm1 is loaded into memory but not displayed until the UserForm1.Show method is called. Between the Load statement and the Show method, you can initialize controls, set properties, or perform other tasks.
Points to Remember
- Memory Allocation: When you use Load, the form is loaded into memory, but it isn’t visible. This means the form’s Initialize event will occur, but the Activate event will not occur until the form is shown.
- Unloading Forms: After using the form, you can unload it from memory using the Unload statement, like Unload UserForm1.
- Efficiency: Preloading a form can improve the user experience by reducing the time it takes for the form to appear when it’s shown. This is particularly useful for forms that require time to initialize.
- Multiple Instances: If you want to create multiple instances of a form, you should declare a variable with the form’s type, use Set to create a new instance, and then load the new instance.
Example with Multiple Instances
Dim anotherForm As UserForm1
Set anotherForm = New UserForm1
Load anotherForm
' Initialize and show the form
anotherForm.Show
In this scenario, anotherForm is a separate instance of UserForm1. This allows you to work with multiple forms of the same type simultaneously, each with its own properties and controls.