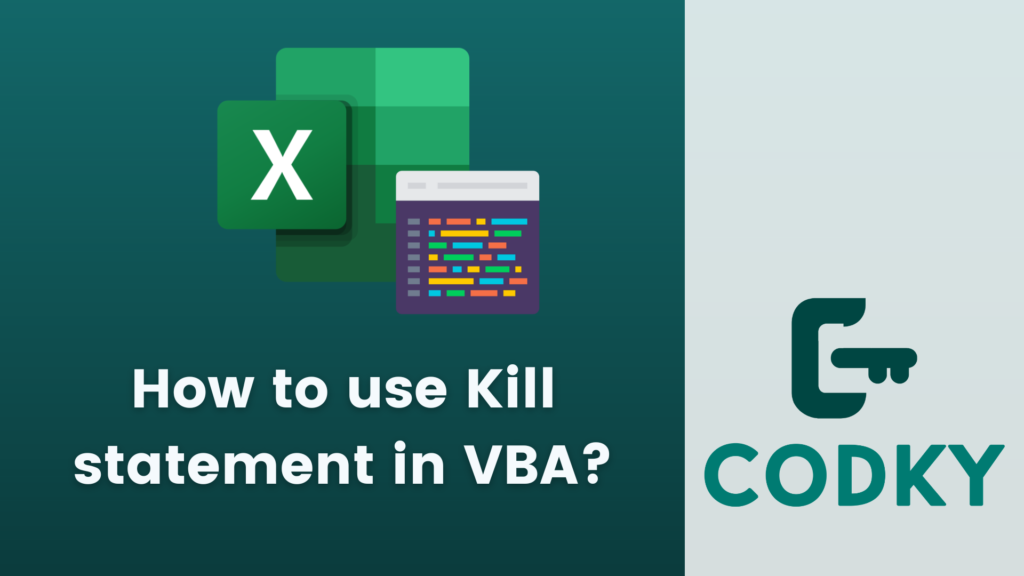
Contents
The Kill statement in VBA (Visual Basic for Applications) is used to delete files from your system. It’s a powerful command that must be used with caution, as it will permanently remove the specified files. Here’s how you can use the Kill statement in VBA:
Basic Usage
VBA
Kill filePath
filePath: This is the path of the file you want to delete. It can include a full path, a relative path, or just the file name if the file is in the same directory as the Excel workbook.
Example
VBA
Sub DeleteFile()
Dim filePath As String
filePath = "C:\path\to\your\file.txt" ' Specify the file path
On Error Resume Next ' In case the file does not exist
Kill filePath
If Err.Number <> 0 Then
MsgBox "File not found or unable to delete.", vbExclamation
Else
MsgBox "File deleted successfully.", vbInformation
End If
End Sub
In this example:
- The filePath variable is set to the full path of the file you want to delete.
- The On Error Resume Next statement is used to handle the case where the file does not exist or cannot be deleted (for example, if it’s open in another program).
- The Kill command attempts to delete the file.
- The If Err.Number <> 0 Then checks if there was an error in deleting the file, and if so, it displays an appropriate message.
Important Points
- Use with Caution: Since Kill permanently deletes files, be very careful with the file paths you specify. Accidental deletion of important files can occur.
- Error Handling: Always include error handling when using Kill to manage situations where the file might not exist or is locked by another process.
- Wildcards: You can also use wildcards with Kill. For example, Kill “C:\path\to\folder\*.txt” will delete all .txt files in the specified folder.
- Permission Issues: Be aware that attempting to delete a file that is open in another application or for which you don’t have sufficient permissions will result in an error.
- File Recovery: Deleted files might not always be recoverable from the Recycle Bin, depending on the system settings and the way VBA interacts with the file system.
Always double-check your file paths and ensure that your code includes adequate error handling when using the Kill statement.