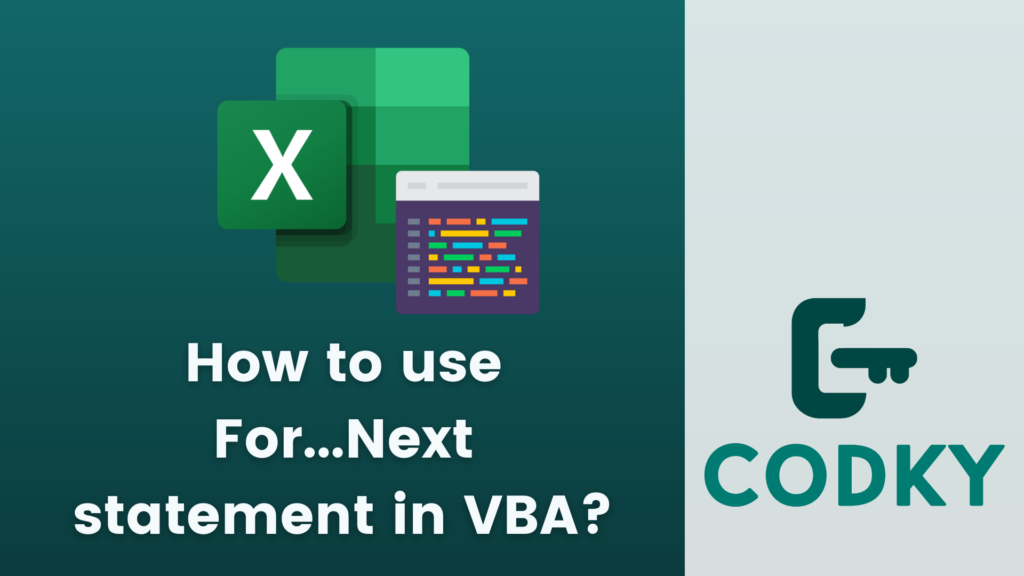
Contents
The For…Next statement in VBA (Visual Basic for Applications) is a control flow statement that allows you to run a block of code a specific number of times. It’s particularly useful when you know in advance how many times you want to execute the statements in the loop.
Syntax:
VBA
For counter = start To end [Step step]
[statements]
[Exit For]
[statements]
Next [counter]
- counter: This is a variable used as the loop counter.
- start: The initial value of the counter.
- end: The final value of the counter.
- Step step: Optional. The value by which the counter is incremented each time through the loop. If omitted, the step is assumed to be 1.
- statements: The lines of code that you want to execute for each iteration of the loop.
- Exit For: Optional. It immediately exits the For loop.
Example:
Suppose you want to loop through numbers 1 to 10 and print each number in the Immediate Window (in the VBA editor).
VBA
Dim i As Integer
For i = 1 To 10
Debug.Print i
Next i
In this example, the variable i starts at 1 and increments by 1 each loop until it reaches 10.
Using Step:
You can use the Step keyword to change the increment. For example, to count backwards from 10 to 1:
VBA
For i = 10 To 1 Step -1
Debug.Print i
Next i
Or to increment by 2:
VBA
For i = 1 To 10 Step 2
Debug.Print i
Next i
Key Points:
- Initialization and Increment: The loop counter is automatically initialized and incremented.
- Deterministic Iterations: Use For…Next when the number of iterations is known before entering the loop.
- Exiting the Loop: You can use Exit For to exit from the loop prematurely, often based on a condition within the loop.
- Flexibility with Step: The Step keyword allows for various increments and even negative steps for decrementing.
Remember that the For…Next loop is a fundamental construct in VBA and understanding it is crucial for writing effective VBA code.