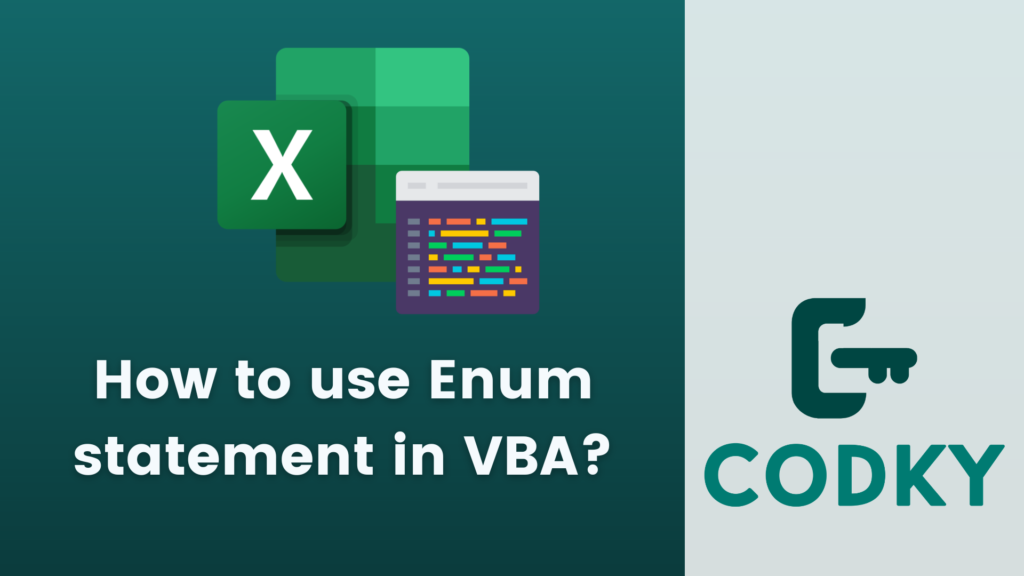
Contents
Using an Enum (short for Enumeration) statement in VBA (Visual Basic for Applications) is a great way to make your code more readable and maintainable. An Enum is essentially a custom data type that you define, which contains a set of related constants. Here’s a step-by-step guide on how to use an Enum statement in VBA:
Define the Enum: You start by defining the Enum with the Enum keyword, followed by a name for your Enumeration. This is usually done at the top of a module, before any procedures.
Enum Season
Spring
Summer
Autumn
Winter
End Enum
Assigning Values: By default, the first item in an Enum has a value of 0, and each subsequent item increments by 1. However, you can manually assign values to the items.
Enum Season
Spring = 1
Summer = 2
Autumn = 3
Winter = 4
End Enum
Using Enum in Code: You can now use the Enum in your procedures and functions as a data type. This makes your code more readable, as it’s clear what the set of allowable values are.
Sub ShowSeason(season As Season)
Select Case season
Case Season.Spring
MsgBox "It's Spring!"
Case Season.Summer
MsgBox "It's Summer!"
Case Season.Autumn
MsgBox "It's Autumn!"
Case Season.Winter
MsgBox "It's Winter!"
End Select
End Sub
Calling a Procedure with Enum: When calling a procedure that uses an Enum, you can pass in the Enum values directly.
Sub Test()
ShowSeason Season.Summer
End Sub
IntelliSense Support: When you use an Enum in your code, VBA’s IntelliSense will provide you with a dropdown list of the Enum’s values, which can help prevent errors and speed up coding.
Scope of Enum: By default, Enum definitions are public within the module they’re declared in. If you want to restrict their scope to the module, use the Private keyword.
Private Enum Season
Spring
Summer
Autumn
Winter
End Enum
Strong Typing: Enums provide strong typing, which means that you can set procedure arguments to be of a specific Enum type, reducing errors and making your code more robust.
Using Enum effectively makes your VBA code more structured, easier to understand, and reduces the likelihood of invalid values being used.