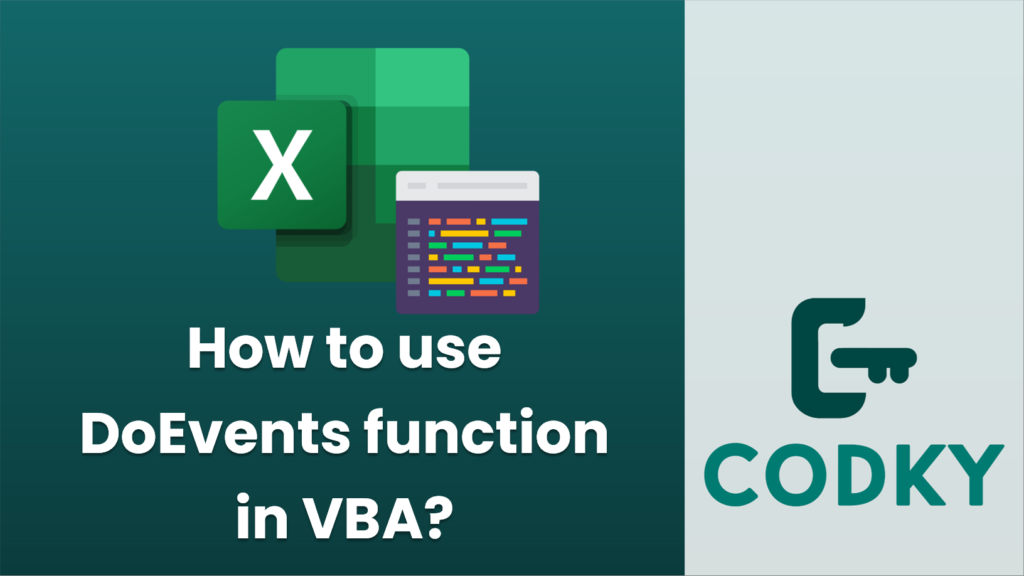
Contents
DoEvents in Visual Basic for Applications (VBA) is a function that allows the operating system to process other events, such as user interactions, messages from other applications, or updates to the user interface, while a procedure is running. This can be especially useful in long-running loops or operations that might make the application seem unresponsive.
To use DoEvents within a VBA code, simply call the function at a point where you want to yield control to the operating system. Here’s a basic example of its usage:
Sub LongRunningProcess()
Dim i As Long
For i = 1 To 1000000 ' Simulating a long process
' Your long-running code here
' Periodically call DoEvents to allow for UI refreshes and other events
If i Mod 1000 = 0 Then ' Call DoEvents every 1000 iterations
DoEvents
End If
Next i
End Sub
In this example, DoEvents is called every 1000 iterations of the loop. This allows Excel to update the UI and respond to user events, such as clicks or keypresses.
However, there are some important things to be mindful of when using DoEvents
- Performance: Frequent calls to DoEvents can slow down your code since it takes time to process the pending events.
- User Interactions: DoEvents allows the user to interact with the application while the code is running, which can sometimes lead to unexpected behavior if they interact with UI elements that trigger other code.
- Breakability: When you use DoEvents, users may be able to invoke other macros or close the application, which can cause errors if your code is not designed to handle such interruptions.
- Reentrancy: Calling DoEvents could potentially lead to reentrant scenarios where event handlers get triggered that could call into the same routine again, possibly causing a stack overflow or logic errors.
Always test your code thoroughly when you use DoEvents to ensure it behaves as expected with regards to user interaction and other event processing. Use it judiciously and understand the implications it can have on the execution and responsiveness of your VBA procedures.