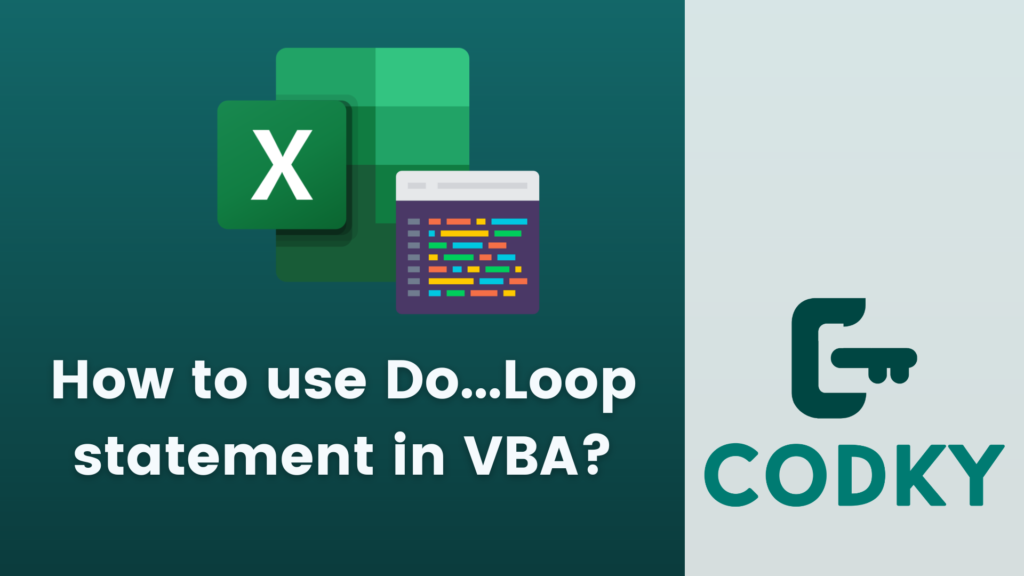
Contents
The Do…Loop statement in VBA (Visual Basic for Applications) is a control structure used to repeat a block of code an indefinite number of times, until a specified condition is met. There are various forms of the Do…Loop statement, each serving different purposes depending on how you want to evaluate the condition and when you want the loop to terminate.
Basic Syntax
The Do…Loop has four basic forms:
Do While…Loop: Repeats the loop as long as the condition is True.
Do While condition
' Code to be executed
Loop
Do…Loop While: Executes the loop at least once, then repeats as long as the condition is True.
Do
' Code to be executed
Loop While condition
Do Until…Loop: Repeats the loop until the condition becomes True.
Do Until condition
' Code to be executed
Loop
Do…Loop Until: Executes the loop at least once, then repeats until the condition becomes True.
Do
' Code to be executed
Loop Until condition
Examples
Do While…Loop Example:
Dim counter As Integer
counter = 0
Do While counter < 5
MsgBox "Counter is: " & counter
counter = counter + 1
Loop
This loop will continue as long as counter is less than 5.
Do…Loop While Example:
Dim counter As Integer
counter = 0
Do
counter = counter + 1
MsgBox "Counter is: " & counter
Loop While counter < 5
This loop will execute at least once and continue until counter becomes 5 or greater.
Do Until…Loop Example
Dim counter As Integer
counter = 0
Do
counter = counter + 1
MsgBox "Counter is: " & counter
Loop Until counter = 5
This loop will execute at least once and will terminate when counter equals 5.
Points to Consider
- Avoiding Infinite Loops: Always ensure that the loop has a clear exit condition to prevent infinite loops. An infinite loop can cause Excel to freeze or crash.
- Incrementing/Decrementing Variables: When using counters or variables to control the loop, make sure they are properly incremented/decremented within the loop.
- Performance: Be cautious with the conditions used in loops, as poorly designed loops can lead to performance issues.
Using Do…Loop statements effectively can help you perform repetitive tasks efficiently in VBA, but they require careful handling to ensure they work as intended and do not lead to program hang-ups.