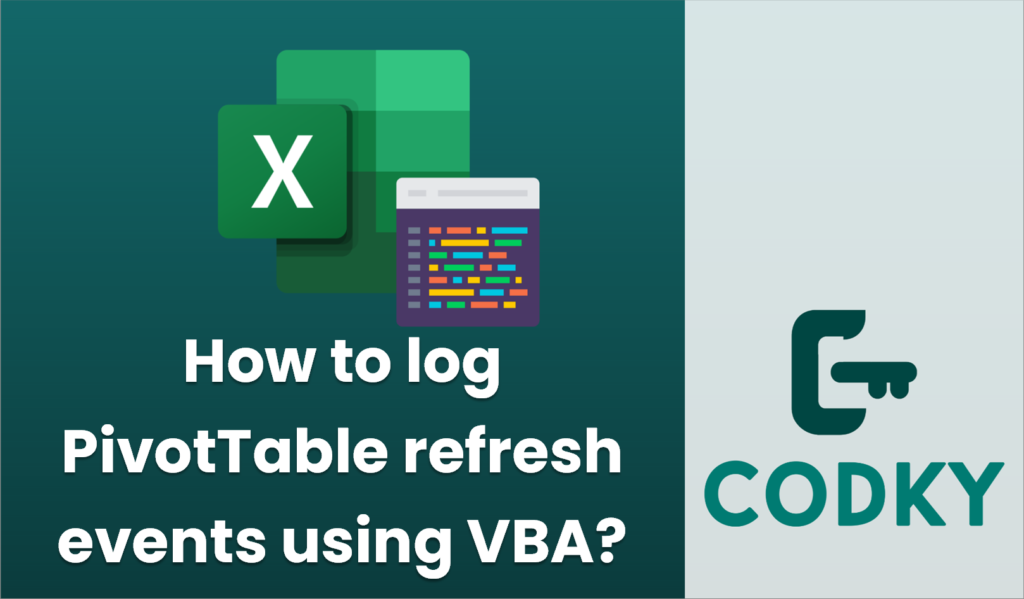
To log PivotTable refresh events using VBA, you’ll need to make use of the `Workbook` or `Worksheet` events to capture when a PivotTable is refreshed. Specifically, you can use the `PivotTableUpdate` event, which triggers whenever a PivotTable in a worksheet is updated. Below is a step-by-step guide on how to set this up:
Private Sub Worksheet_PivotTableUpdate(ByVal Target As PivotTable)
' Log to Immediate Window
Debug.Print "PivotTable '" & Target.Name & "' was updated at " & Now
' Alternatively, log to a text file
Dim logFilePath As String
logFilePath = "C:pathtoyourlogfile.txt" ' Change this path as necessary
Dim logFile As Integer
logFile = FreeFile
Open logFilePath For Append As #logFile
Print #logFile, "PivotTable '" & Target.Name & "' was updated at " & Now
Close #logFile
End Sub
- Open the VBA Editor:
- Press `ALT + F11` to open the Visual Basic for Applications editor.
- Access the Worksheet Module:
- In the Project Explorer window, find the worksheet that contains the PivotTable you want to monitor. Double-click on the worksheet name to open its code window.
- Add the PivotTableUpdate Event:
- In the code window for the worksheet, you need to add a `PivotTableUpdate` event procedure. This event will trigger every time a PivotTable in that particular worksheet is updated. Here is an example of how you can handle this event and log to the Immediate Window or a log file:
- Customize the Log Location:
- Ensure you update the `logFilePath` variable with the correct path where you want to save your log file. Make sure you have permission to write to this location.
- Test the Setup:
- Refresh the PivotTable manually or through some automation to see if the event captures and logs the refresh event as expected.
- Considerations:
- If you have multiple PivotTables across different sheets, you may need to add similar code to each relevant worksheet module or extend the logic to handle multiple situations as necessary.
- Saving and Enabling Macros:
- Remember to save your workbook as a macro-enabled file (`.xlsm`). Also, ensure that macros are enabled when you open the workbook to allow the event code to run.
By following these steps, you can successfully log PivotTable refresh events in Excel using VBA, either to a log file or to the Immediate Window for debugging purposes.