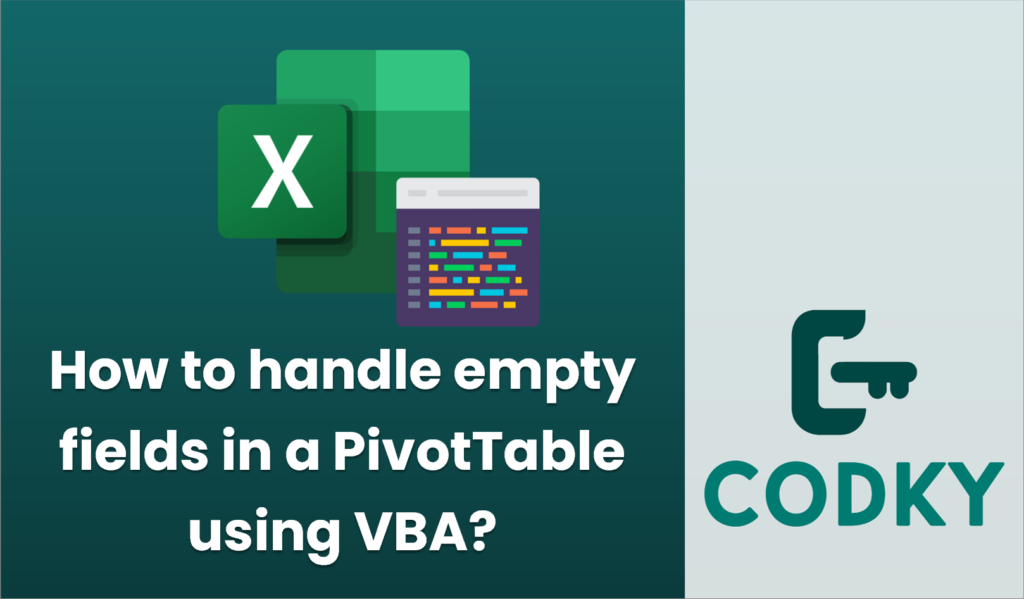
Handling empty fields in a PivotTable using VBA involves checking for these empty values and possibly replacing them with a placeholder or a specific value that makes more sense in your context. Below is a guide on how to achieve this using VBA:
Sub HandleEmptyFieldsInPivotTable()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
Dim pi As PivotItem
Dim dataRange As Range
Dim cell As Range
Dim dbEmpty As Boolean
' Set the worksheet and the PivotTable
Set ws = Worksheets("Sheet1") ' change to your sheet name
Set pt = ws.PivotTables("PivotTable1") ' change to your PivotTable name
' Loop through each row field to handle empty row labels
For Each pf In pt.RowFields
For Each pi In pf.PivotItems
If pi.Value = "(blank)" Then ' Assuming "(blank)" is shown for empty fields
pi.Value = "N/A" ' Replace with desired text
End If
Next pi
Next pf
' Loop through each data cell to handle empty values
Set dataRange = pt.DataBodyRange
If Not dataRange Is Nothing Then
For Each cell In dataRange
If IsEmpty(cell) Or Trim(cell.Value) = "" Then
cell.Value = 0 ' Replace with 0 or desired value
End If
Next cell
End If
MsgBox "Empty fields have been handled.", vbInformation
End Sub
- Open the VBA Editor: Press `ALT + F11` in Excel to open the VBA editor.
- Insert a Module: Right-click on any existing modules or on “VBAProject” and choose `Insert` > `Module` to create a new module if necessary.
- VBA Code: Use the following sample code as a starting point. This script loops through each cell in the PivotTable’s data area and replaces empty values with a placeholder, such as “N/A” or a zero.
- Modify the Code: Adjust the `Worksheets(“Sheet1”)` and `PivotTables(“PivotTable1”)` sections with the actual names of your worksheet and PivotTable.
- Run the Code: Press `F5` to run the script or run it from the Excel window by using a button or shortcut linked to the macro.
Additional Considerations:
- Handling Different Types of Empty Cells: You may want to handle different empty cell representations differently, such as `Null`, `””` (an empty string), or even specific text like `(blank)`. Customize the conditional checks based on your data.
- Error Handling: Consider adding error handling to manage any run-time errors that might occur, especially if your PivotTable or data range specifications are incorrect.
- User Notification: The `MsgBox` is a simple way to notify the user that the process is complete, but you could expand this to provide more detailed feedback.
This script assumes you know the names of your sheets and PivotTables and requires appropriate adjustments on those lines. This approach should help maintain the integrity of analysis by ensuring that missing or empty data fields are appropriately tagged or filled in.