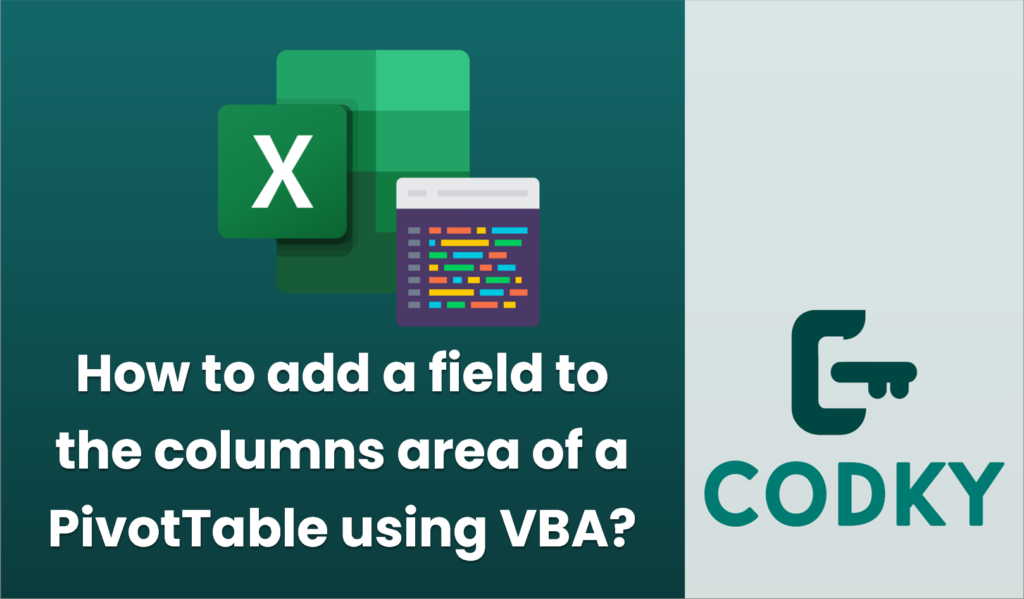
To add a field to the columns area of a PivotTable using VBA, you’ll need to access the `PivotTable` object and then use the `PivotFields` collection to manipulate the fields. Let’s assume you already have a PivotTable created in your worksheet. Here’s a step-by-step guide along with an example code to add a field to the columns area:
- Identify the PivotTable: Determine the name or index of the PivotTable you want to modify. The PivotTable is usually on a particular worksheet.
- Determine the Field: Decide which field from your source data you want to add to the columns area of the PivotTable.
- Write the VBA Code: Use VBA to reference the correct PivotTable and add the desired field to the columns area.
Below is a sample VBA code to achieve this:
Sub AddFieldToColumns()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
' Specify the worksheet containing the PivotTable
Set ws = ThisWorkbook.Worksheets("Sheet1") ' Change "Sheet1" to your worksheet name
' Specify the PivotTable
Set pt = ws.PivotTables("PivotTable1") ' Change "PivotTable1" to your PivotTable name
' Specify the field that you want to add to the Columns area
Set pf = pt.PivotFields("FieldName") ' Replace "FieldName" with the actual field name
' Add the field to the Columns area
With pt
.ColumnFields.Add pf
End With
End Sub
Key Points to Remember:
- Worksheet and PivotTable Names: Ensure you have the correct names for the worksheet and PivotTable.
- Field Name: The `FieldName` should match exactly as it appears in the source data.
- VBA Environment: You need to run this VBA code from the VBA editor (accessible by pressing `ALT + F11` in Excel).
Troubleshooting:
- If you encounter an error, double-check the worksheet and PivotTable names.
- Ensure that the field name exists in the PivotTable source data.
- Before running the VBA code, ensure macros are enabled in Excel, and save any important work since VBA changes cannot be undone with the Undo button.
This VBA script is a simple and efficient way to automate modifications and enhancements to your PivotTable reports in Excel.