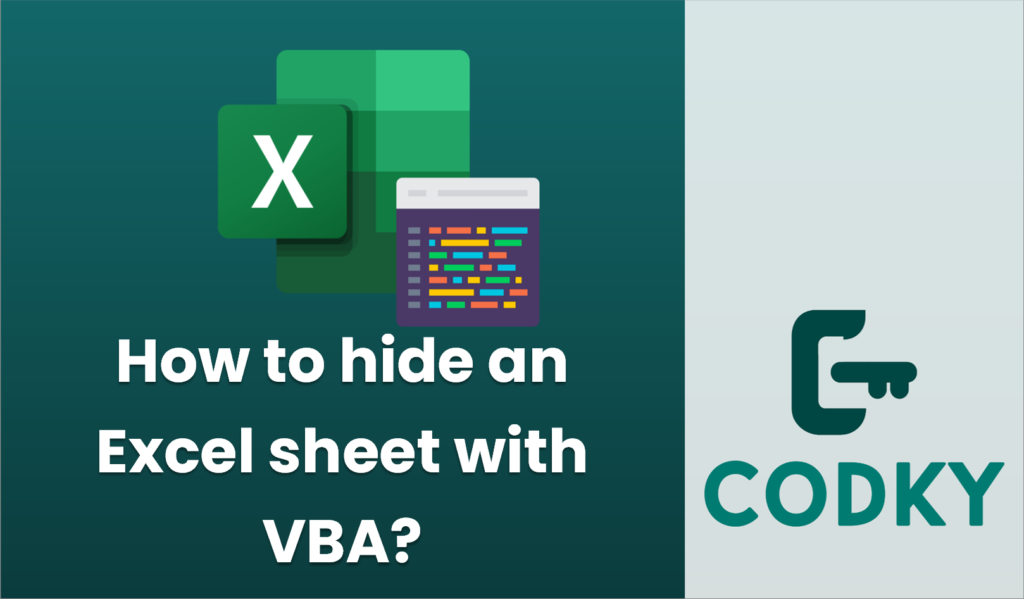
To hide an Excel sheet using VBA, you can use the `Visible` property of the `Worksheet` object. You have the option to make the sheet hidden or very hidden. Here’s how you can do both:
- Make a Sheet Hidden: The sheet can be hidden, but still visible in the “Unhide” dialog box under the “View” tab in Excel.
- Make a Sheet Very Hidden: The sheet is hidden and does not appear in the “Unhide” dialog box, making it more secure.
Here’s a step-by-step guide to use VBA for both:
Open the VBA Editor
- Press `Alt` + `F11` to open the VBA Editor.
- In the VBA Editor, locate the workbook and sheet that you want to hide.
Hide a Sheet
To just hide a sheet and allow it to be unhidden through the Excel interface:
Sub HideSheet()
ThisWorkbook.Sheets("SheetName").Visible = xlSheetHidden
End Sub
Replace `”SheetName”` with the name of the sheet you want to hide.
Make a Sheet Very Hidden
To make a sheet very hidden, so it does not appear in the “Unhide” dialog box:
Sub VeryHideSheet()
ThisWorkbook.Sheets("SheetName").Visible = xlSheetVeryHidden
End Sub
Again, replace `”SheetName”` with the actual name of your worksheet.
Run the VBA Code
- Ensure the correct macro is selected.
- Press `F5` to run the macro, or click the “Run” button in the VBA Editor.
Unhide Very Hidden Sheet
To unhide a very hidden sheet, use the following code:
Sub UnhideSheet()
ThisWorkbook.Sheets("SheetName").Visible = xlSheetVisible
End Sub
Remember, making a sheet “Very Hidden” is useful for security, but anyone with access to the VBA Editor can change it back. If securing sensitive data, consider password-protecting your VBA project.