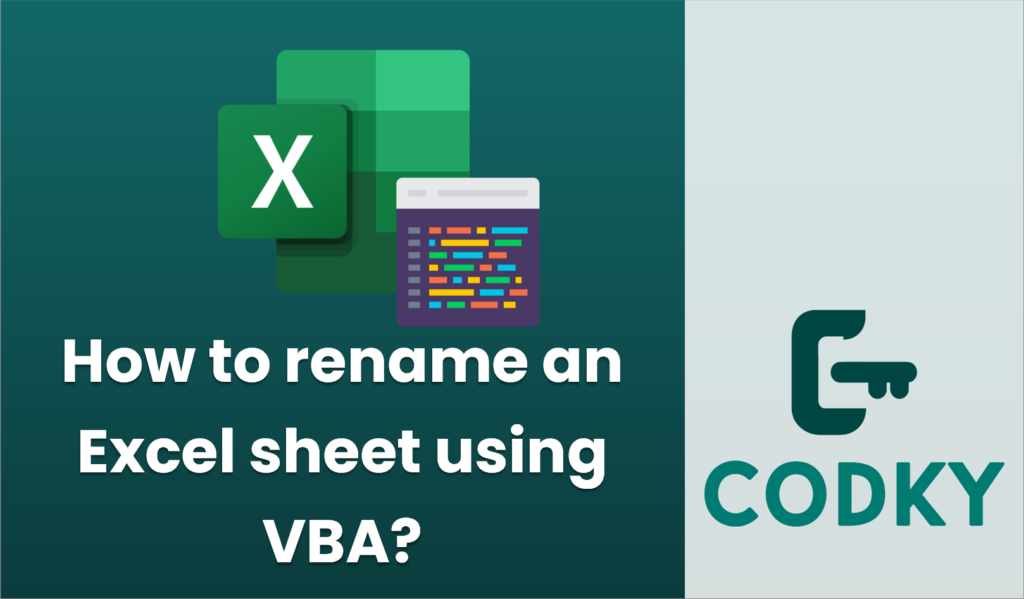
You can rename an Excel sheet using VBA by referring to the `Sheets` or `Worksheets` collection. You’ll need to specify the sheet you want to rename and then change its `Name` property. Here’s an example of how to do it:
Sub RenameSheet()
' This example renames the first sheet to "NewSheetName"
Dim sheetIndex As Integer
Dim newSheetName As String
' Specify the index of the sheet to rename
sheetIndex = 1
' Specify the new name for the sheet
newSheetName = "NewSheetName"
' Rename the sheet
Sheets(sheetIndex).Name = newSheetName
' Alternatively, you can refer to the sheet by its current name
' Sheets("OldSheetName").Name = newSheetName
End Sub
- Open the Visual Basic for Applications (VBA) editor:
- Press `ALT` + `F11` in Excel to open the VBA editor.
- Insert a new module:
- Click on `Insert` in the menu and then select `Module`. This will create a new module where you can write your VBA code.
- Write the VBA code:
- To rename an Excel sheet, you can use the following code. Make sure to adjust the example to fit your needs.
- Run the VBA code:
- You can run this macro by pressing `F5` while the code is open or by going back to Excel, opening the “Macros” dialog (`ALT` + `F8`), selecting `RenameSheet`, and clicking `Run`.
Important Notes:
- Error handling: Make sure the new sheet name does not conflict with any existing sheet names, as Excel does not allow duplicate sheet names. Also, ensure that the new name follows Excel’s sheet naming rules (e.g., it cannot be more than 31 characters, contain certain characters like `:`, “, `/`, `?`, `*`, etc.).
- Index vs. Name: You can refer to a sheet by its index or current name. If you use its name, ensure that it matches exactly.
- Nonexistent sheets: Ensure that the specified sheet index or name exists to avoid runtime errors. You might want to add error handling for more robust scripts.