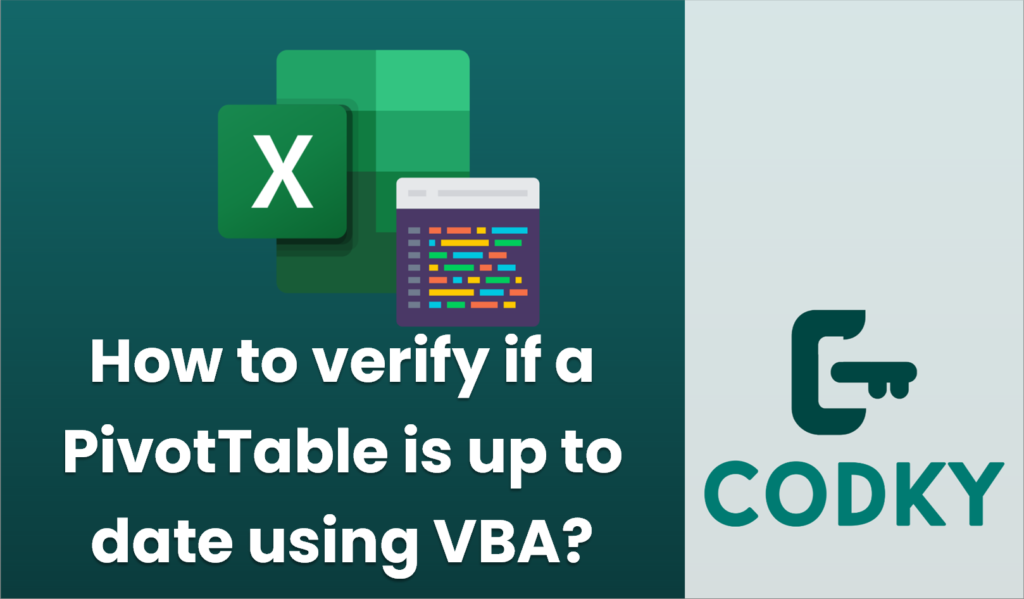
To verify if a PivotTable is up-to-date using VBA, you need to check if the data source (typically a range or a table) has been modified since the last refresh of the PivotTable. A straightforward way to do this is to compare the last refresh time of the PivotTable with a known timestamp that indicates when the data was last updated. Unfortunately, Excel does not provide a built-in feature to track when data in a range was last modified, so you may need to manually update a timestamp cell each time data changes.
Here’s a simple approach using VBA to verify if the PivotTable is up-to-date:
- Timestamp Cell: Add a cell in your worksheet that records the last modified time of your data range. This cell needs to be manually updated whenever the data changes.
- VBA Code: Use VBA to compare the last refresh time of the PivotTable with the timestamp.
Here’s an example VBA script that checks if a PivotTable is up-to-date:
Sub CheckPivotTableUpToDate()
Dim ws As Worksheet
Dim pt As PivotTable
Dim timestampCell As Range
Dim dataUpdatedTime As Date
Dim lastRefreshTime As Date
' Set worksheet and PivotTable
Set ws = ThisWorkbook.Worksheets("Sheet1") ' Change to your sheet name
Set pt = ws.PivotTables("PivotTable1") ' Change to your PivotTable name
' Set the cell where the last data update timestamp is stored
Set timestampCell = ws.Range("A1") ' Change to your timestamp cell
' Get the data updated time from the cell
dataUpdatedTime = timestampCell.Value
' Get the last refresh time of the PivotTable
lastRefreshTime = pt.RefreshDate
' Compare the times
If lastRefreshTime >= dataUpdatedTime Then
MsgBox "The PivotTable is up to date.", vbInformation
Else
MsgBox "The PivotTable is not up to date.", vbExclamation
End If
End Sub
Steps to Use the Code:
- Ensure that you have a cell that you update with the current timestamp each time your data changes. For example, you can manually enter this or use a VBA script triggered by a data change event to update it automatically.
- The code assumes the timestamp is stored in cell `A1`. You need to change the `ws`, `pt`, and `timestampCell` variables according to your worksheet, PivotTable, and timestamp cell locations.
- Run this script to check the status. It will display a message box indicating whether the PivotTable is up-to-date.
Notes:
- Automating the update of the timestamp could involve monitoring the worksheet for changes and updating the timestamp accordingly. This can be complex, depending on how often and by what means your data is updated.
- This method provides a basic approach. In practice, you might need more sophistication depending on your workflow. For instance, if your data comes from external sources, you may have to integrate additional logic to track changes.