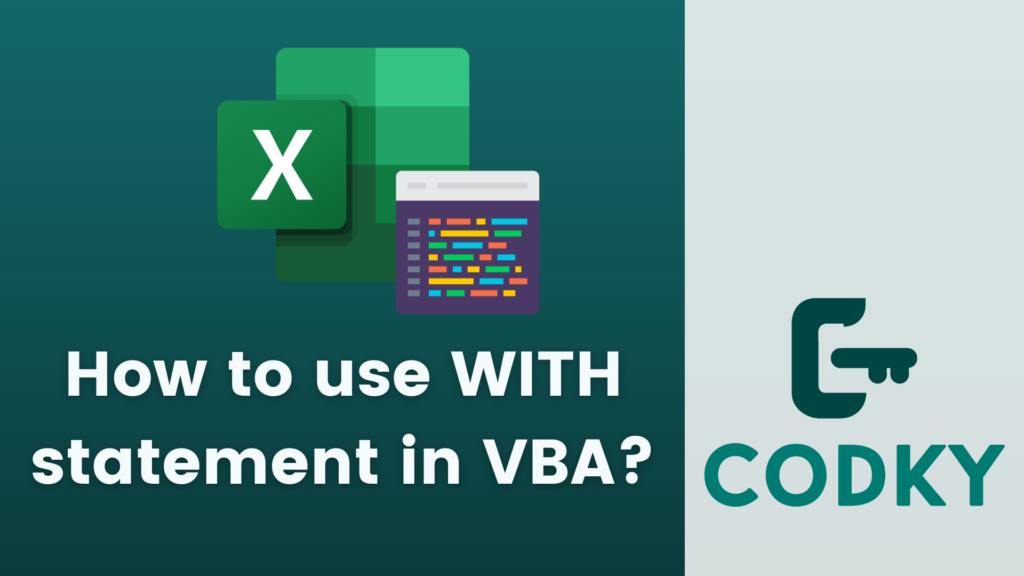
Basic Syntax
VBA
object: This is the object you want to perform multiple actions on.
With object
' Statements that use properties or methods of the object
End With
Example Usage
Suppose you’re working with an Excel worksheet and you want to format a range of cells. Without With, you might write:VBA
With the With statement, this can be simplified to:
Worksheets("Sheet1").Range("A1").Font.Bold = True
Worksheets("Sheet1").Range("A1").Font.Italic = True
Worksheets("Sheet1").Range("A1").Font.Size = 12
VBA
Example Usage:
Suppose you're working with an Excel worksheet and you want to format a range of cells. Without With, you might write:
VBAWorksheets("Sheet1").Range("A1").Font.Bold = True
Worksheets("Sheet1").Range("A1").Font.Italic = True
Worksheets("Sheet1").Range("A1").Font.Size = 12
Nested With Statements
You can nest With statements when working with objects that contain other objects. For example:VBA
With Worksheets("Sheet1")
With .Range("A1")
.Value = "Test"
.Font.Name = "Arial"
End With
End With
Improving Readability and Efficiency
- The With statement makes your code more readable by eliminating the need to repeat the object reference for each property or method call.
- It can also improve the efficiency of your code, particularly when the object reference is a complex expression that VBA would otherwise need to evaluate multiple times.