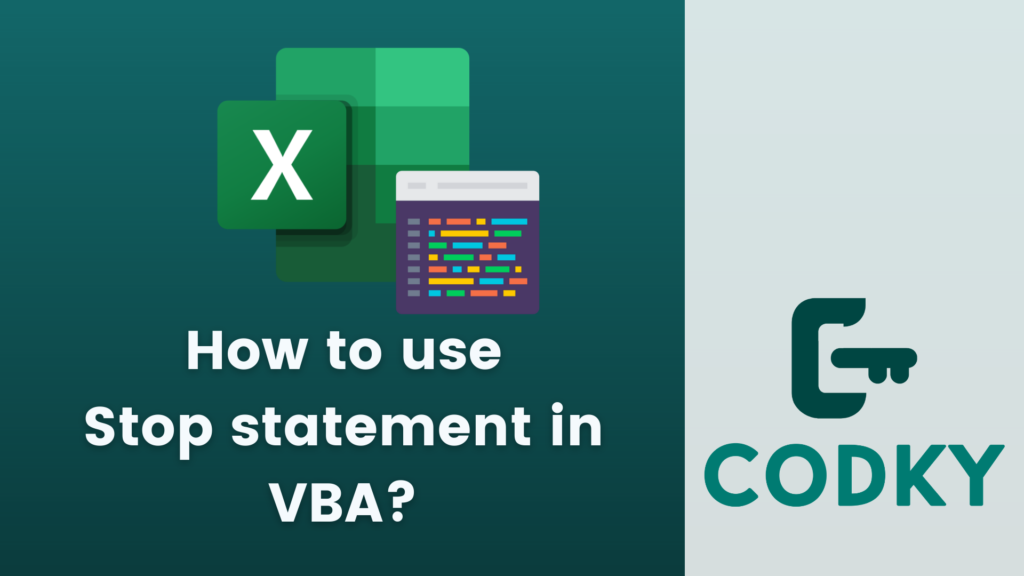
Contents
The Stop statement in VBA (Visual Basic for Applications) is used to pause the execution of a program in the development environment. When a Stop statement is encountered, the program enters break mode. This is particularly useful for debugging purposes, as it allows you to examine the values of variables, the flow of the program, and to step through the code line by line.
Here’s how to use the Stop statement:
Basic Usage:
Simply insert the Stop statement in your code where you want the execution to pause. For example:
Sub MyMacro()
' Some code
Stop
' More code
End Sub
Example for Debugging
You might use Stop to check the state of your variables or the flow of your program at a specific point. For instance:
Dim counter As Integer
For counter = 1 To 10
If counter = 5 Then
Stop ' Execution will pause when counter equals 5
End If
' Some other code
Next counter
Temporary Use During Development:
The Stop statement is often used temporarily during the development and debugging phase. It’s generally a good practice to remove or comment out Stop statements from your final code, as they are not needed in a production environment and can inadvertently interrupt the program.
Using Breakpoints Instead
Instead of using the Stop statement, you can also set breakpoints in the VBA IDE by clicking in the margin next to the code line. Breakpoints can be more convenient than Stop statements because they can be easily toggled on and off without modifying the code.
Interaction with Error Handling
When used within a procedure that has error handling code (On Error Resume Next or On Error GoTo Label), the Stop statement will still cause the program to enter break mode, which can be useful for debugging error handling routines.
Limitations
Stop statements have no effect when a VBA program is running outside the development environment (like when a macro is executed by a user in a deployed application). In such cases, the program will ignore the Stop statement and continue execution.
Using Stop is a simple and effective way to pause execution and can be a valuable tool in the debugging process, helping you to understand and fix issues in your VBA code.