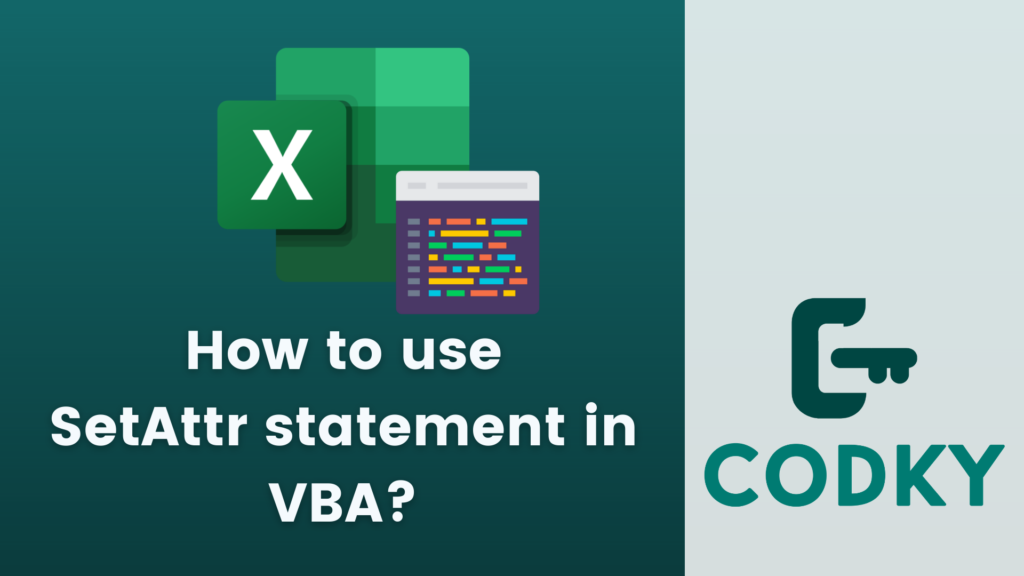
Contents
In VBA (Visual Basic for Applications), the SetAttr statement is used to set the attribute of a file or directory. File attributes include settings like read-only, hidden, system file, and so on. You can use this statement to modify these attributes programmatically.
Here’s how to use the SetAttr statement:
Basic Syntax
SetAttr pathname, attributes
- pathname: This is a string expression that specifies a file or directory. The path may be absolute or relative.
- attributes: This is a numeric expression that specifies the file attributes to set. It’s a combination of the following intrinsic constants:
- vbNormal (0): Normal (no attributes).
- vbReadOnly (1): Read-only.
- vbHidden (2): Hidden.
- vbSystem (4): System file.
- vbArchive (32): File has changed since last backup. This attribute is often used by backup programs.
Example Usage
SetAttr "C:\MyFolder\MyFile.txt", vbReadOnly
This command sets the file MyFile.txt in MyFolder on the C drive as read-only.
Combining Attributes
You can combine multiple attributes using the Or operator. For example, to set a file as hidden and read-only:
SetAttr "C:\MyFolder\MyFile.txt", vbHidden Or vbReadOnly
Removing Attributes
To remove a specific attribute, you need to first get the current attributes using the GetAttr function, then use bitwise operations to clear the desired attribute. For example, to remove the read-only attribute:
Dim currentAttr As Integer
currentAttr = GetAttr("C:\MyFolder\MyFile.txt")
If (currentAttr And vbReadOnly) = vbReadOnly Then
SetAttr "C:\MyFolder\MyFile.txt", currentAttr And Not vbReadOnly
End If
Error Handling
Make sure the file or directory exists before attempting to set its attributes. If the file or directory does not exist, SetAttr will result in a runtime error.
It’s a good practice to include error handling to deal with potential errors, such as permission issues.
Considerations
- Changing system file attributes should be done cautiously, as it can affect the operating system or application behaviors.
- SetAttr can’t be used to set or clear the vbDirectory attribute. It’s automatically set by the system for directories.
Practical Applications
SetAttr is often used in applications that need to manage file security or visibility, like in custom file managers, backup utilities, or system maintenance tools.
Remember, modifying file attributes, especially for system or hidden files, should be done with care, as it might have unintended consequences on system stability or file accessibility.