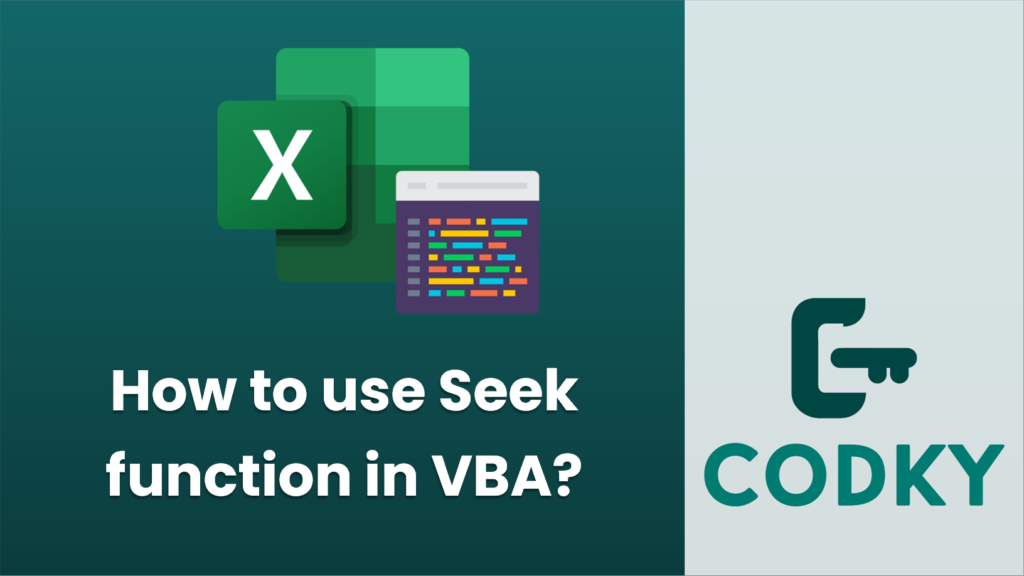
Here’s how to use the Seek function
- Open a file using the Open statement with Binary, Input, or Output mode.
- Use Seek to either retrieve or set the position of the file pointer.
To get the current position:
VBA
Dim fileNumber As Integer
Dim currentPosition As Long
fileNumber = FreeFile() ' Get the next available file number
Open "C:pathtoyourfile.txt" For Binary Access Read As #fileNumber
currentPosition = Seek(fileNumber) ' Retrieve the current position
Close #fileNumber
To set a new position:
VBA
Dim fileNumber As Integer
Dim newPosition As Long
fileNumber = FreeFile() ' Get the next available file number
Open "C:pathtoyourfile.txt" For Binary Access Write As #fileNumber
newPosition = 100 ' Example of the new position you want to set
Seek #fileNumber, newPosition ' Set the new position
' Now you can read from or write to the file at the new position
' ...
Close #fileNumber
Notes
When using Seek to set a new position, if you specify a position beyond the current end-of-file, the file size is increased to accommodate the new position and the intervening bytes are filled with zeros.When you use Seek in conjunction with files opened for Binary access, you can move to any position within the file and read or write bytes from that position.
When you use Seek with files opened for Input or Output, you are dealing with records rather than arbitrary byte positions. It’s important to handle errors when dealing with file operations, so consider using On Error statements to catch any unexpected problems, and always ensure you close files after you’re done with them to avoid locking the file or leaking system resources.