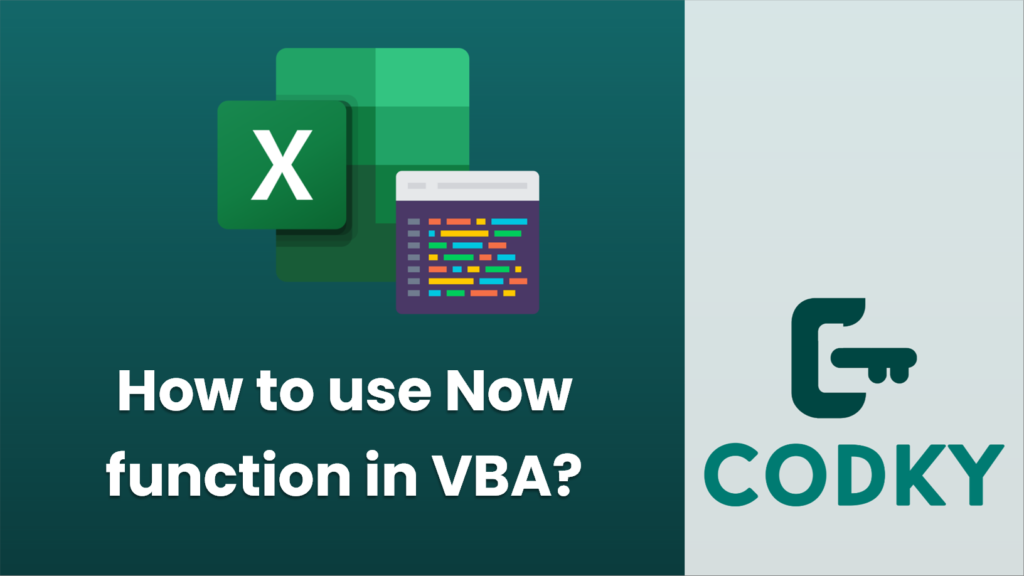
In VBA (Visual Basic for Applications), the `Now` function is used to get the current date and time. The `Now` function does not require any arguments and can be used in your VBA code to insert the current date and time into a cell, variable, or use it for time-stamping and calculations.
Here’s how you can use the `Now` function in VBA:
Sub InsertCurrentDateTime()
' This will insert the current date and time into cell A1 of the active sheet
ActiveSheet.Range("A1").Value = Now
End Sub
Sub GetCurrentDateTime()
Dim currentDateTime As Date
currentDateTime = Now
Debug.Print "The current date and time is: " & currentDateTime
End Sub
Sub CalculateTimeDifference()
Dim startTime As Date
Dim endTime As Date
Dim timeDifference As Double
' Record start time
startTime = Now
' (Your code that performs tasks goes here)
' Record end time
endTime = Now
' Calculate the difference in seconds
timeDifference = (endTime - startTime) * 24 * 60 * 60
Debug.Print "The operation took " & timeDifference & " seconds to complete."
End Sub
Sub ShowCurrentDateTime()
MsgBox "The current date and time is: " & Now
End Sub
- Insert the current date and time into a cell:
- Assign the current date and time to a variable:
- Use the current date and time in a calculation:
- Display a message with the current date and time:
Remember that `Now` returns both the date and time. If you need just the date without the time component, you would use the `Date` function, and for just the time component, use the `Time` function.
To utilize these functions, you can simply call them directly in your VBA procedures wherever you need the current date and time.