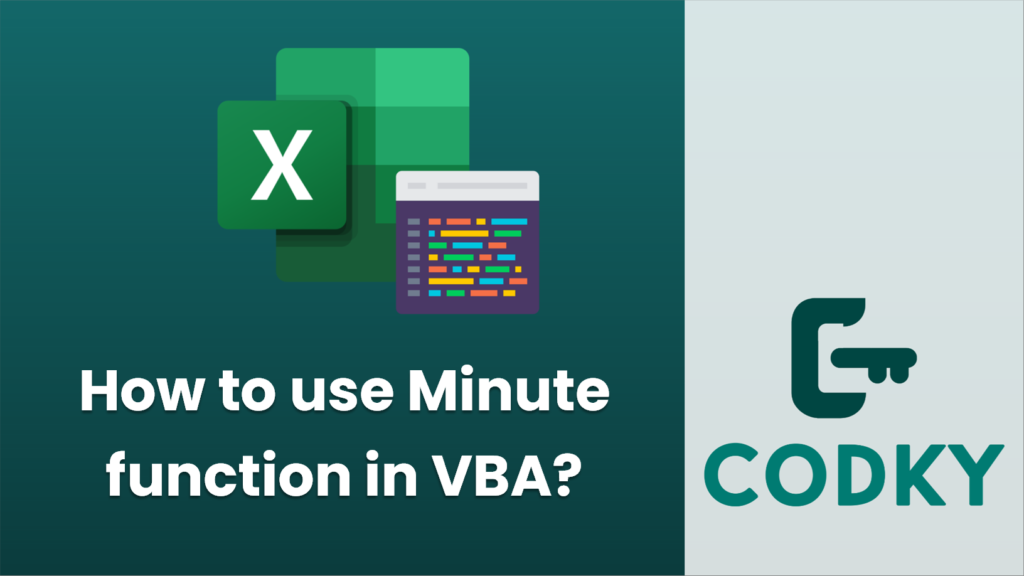
In VBA (Visual Basic for Applications), the `Minute` function is used to retrieve the minute portion from a given time. The time can be specified as a serial date-time code, or it can be passed directly as a string that represents time or a date-time value. The `Minute` function returns an integer value between 0 and 59.
Here’s a basic example of how to use the `Minute` function:
Sub ExampleMinute()
Dim currentTime As Date
Dim minutes As Integer
' Set the current time
currentTime = Now
' Retrieve the minute portion of the current time
minutes = Minute(currentTime)
' Display the minutes in a message box
MsgBox "The current minute is: " & minutes
End Sub
In this code, we’re using the `Now` function to get the current date and time, and then we use the `Minute` function to extract the minutes from the current time. Finally, we display this value in a message box.
If you want to get the minutes from a specific time, not the current time, you could do this:
Sub GetMinutesFromSpecificTime()
Dim specificTime As Date
Dim minutes As Integer
' Specify a time
specificTime = #1:30:45 PM#
' Retrieve the minute portion of the specified time
minutes = Minute(specificTime)
' Display the minutes in a message box
MsgBox "The minute is: " & minutes
End Sub
In this example, we’re using a specific hard-coded time within `##` delimiters to denote a date/time literal in VBA. The `Minute` function then retrieves the minute part of that specific time.
Keep in mind that `Minute` only returns the minute component of the time, if you need the hour or second, you would use the `Hour` or `Second` function respectively.