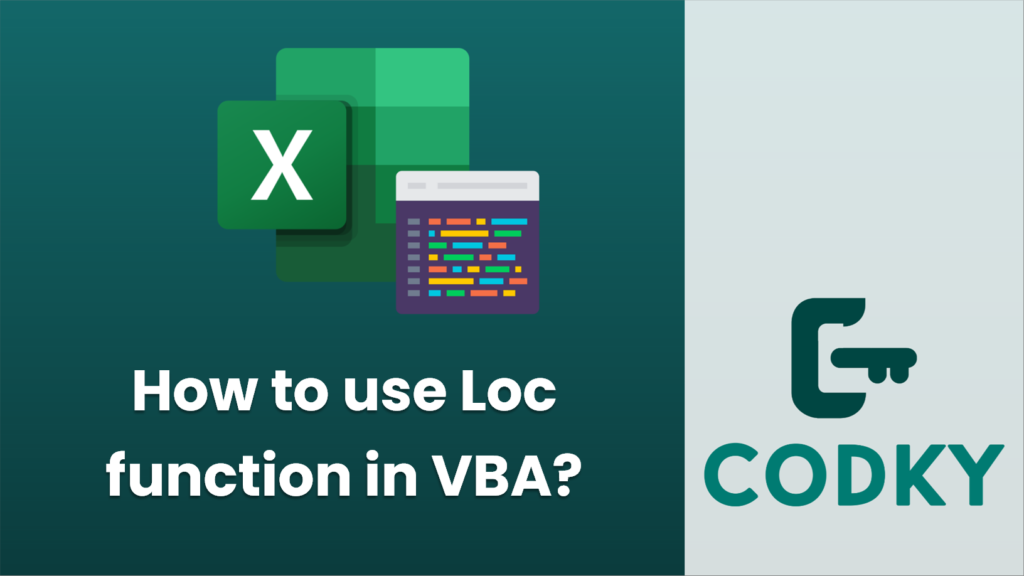
In VBA (Visual Basic for Applications), the `Loc` function is used to obtain the current read/write position within an open file. This is most often used when you are working with files in a binary mode, as `Loc` will return the position as the number of bytes from the start of the file.
Here’s the syntax for the `Loc` function:
position = Loc(fileNumber)
Where `fileNumber` is a required argument that represents the file number you want to get the position for. The file number is the number that is specified when you open the file using the `Open` statement. The result, `position`, is the current position in the file.
Here’s an example of using the `Loc` function:
Sub FilePositionExample()
Dim fileNum As Integer
Dim filePath As String
Dim filePos As Long
' Set the path to the file
filePath = "C:pathtoyourfile.txt"
' Get the next available file number
fileNum = FreeFile()
' Open the file for Binary Access
Open filePath For Binary Access Read As #fileNum
' Do some file read or write operations here
' ...
' Get the current position in the file
filePos = Loc(fileNum)
' Show the position
MsgBox "The current file position is: " & filePos
' Close the file
Close #fileNum
End Sub
When you run this code, it will open the specified file in binary mode, and then use the `Loc` function to find out the current position of the file pointer. Since there are no read or write operations in the example between the opening and the check, it would typically be at the beginning of the file when the `MsgBox` is shown.
Please remember that the `Loc` function is not frequently used for text files, as reading or writing operations with text files move the file pointer in variable-length increments, making `Loc` values for text files less meaningful.
Also, note that `Loc` function works with files opened for Binary, Random, and Input modes, but does not work with files opened for Output or Append.
Make sure that the file exists and you have appropriate permissions to read from the file if you are using the `Loc` function in conjunction with reading file operations.