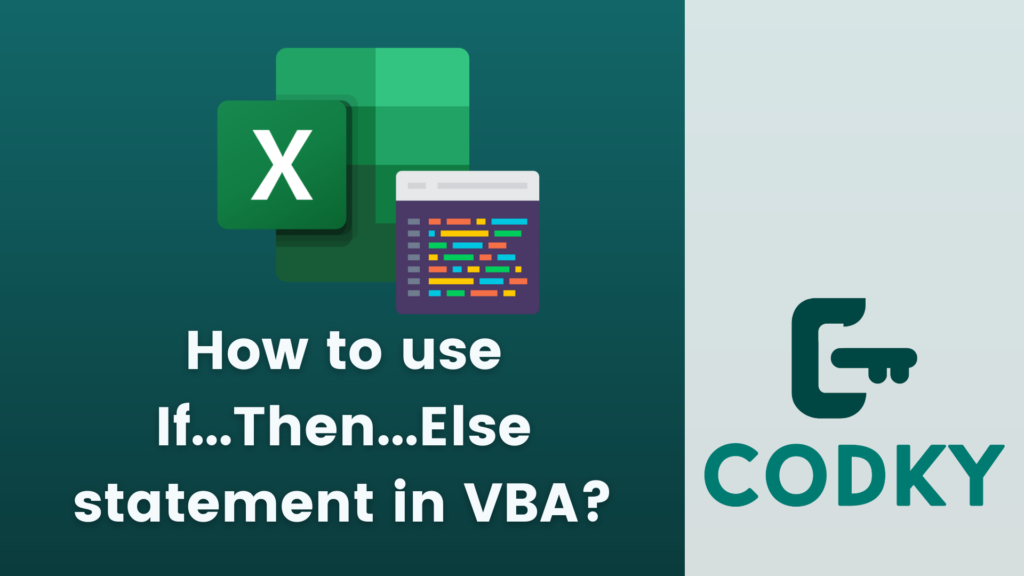
Basic Structure
VBA
If [condition] Then
' Code to execute if condition is True
Else
' Code to execute if condition is False
End If
- [condition]: This is where you put your logical test. If the condition evaluates to True, the first block of code will run; otherwise, the Else block will run.
- Then: Indicates the start of the block of code that executes if the condition is True.
- Else (optional): Starts the block of code that executes if the condition is False.
- End If: Marks the end of the If statement.
VBA
In this example:
Sub CheckAge()
Dim age As Integer
age = 25
If age >= 18 Then
MsgBox "You are an adult."
Else
MsgBox "You are not an adult."
End If
End Sub
- We first declare a variable age and assign it a value of 25.
- The If statement checks if age is greater than or equal to 18.
- If the condition is True (which it is in this case), a message box saying “You are an adult.” will appear.
- If the condition were False, a different message box saying “You are not an adult.” would appear.
- This is a simple demonstration, but If…Then…Else statements can be more complex, including multiple conditions using ElseIf, or nested If statements for more intricate logic.