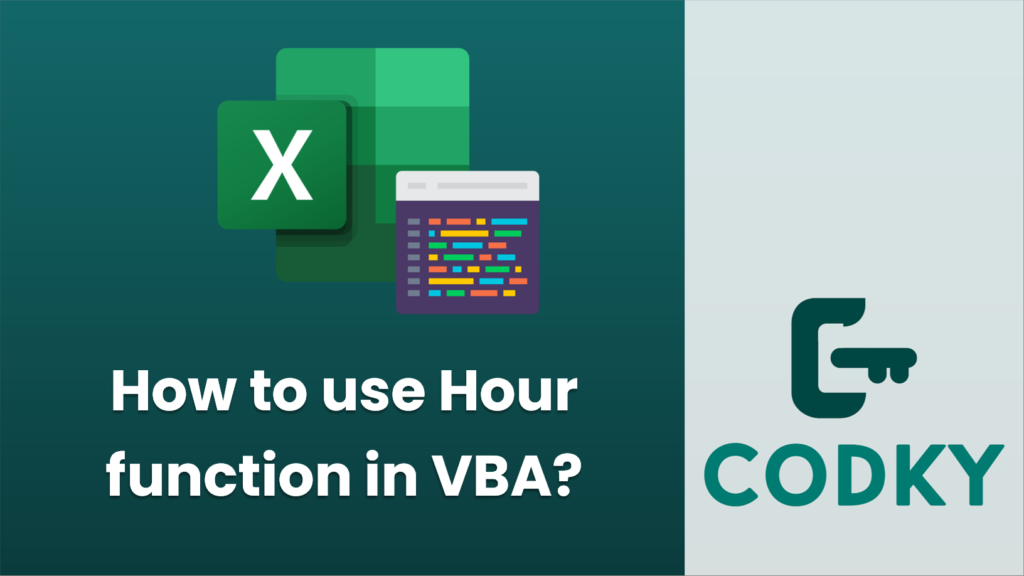
In VBA (Visual Basic for Applications), the `Hour` function is used to extract the hour part from a given time. The function returns an integer value between 0 and 23, representing the hour of the day.
Here’s how you can use the `Hour` function:
- Open the Excel application.
- Press `ALT` + `F11` to open the Visual Basic for Applications editor.
- Insert a new module or use an existing one.
- Write a VBA subroutine or function to utilize the `Hour` function.
Below is an example of a subroutine that uses the `Hour` function:
Sub GetHourExample()
Dim exampleTime As Date
Dim extractedHour As Integer
' Example time
exampleTime = "15:30:25" ' This is 3:30:25 PM.
' Use the Hour function to get the hour part of the time
extractedHour = Hour(exampleTime)
' Display the result in a message box
MsgBox "The hour part of the time is: " & extractedHour
End Sub
In this example, the subroutine `GetHourExample` defines a time which is stored in the `exampleTime` variable. The `Hour` function is then used to extract the hour part of this time, and the result is stored in the `extractedHour` variable. A message box is then used to display the result.
To run this code, you would need to place the cursor inside the subroutine and press `F5` or select the ‘Run’ option from the toolbar.
You can also use the `Hour` function within a function to return the hour part of a time variable that could be passed as an argument:
Function GetHourOfTime(timeVal As Date) As Integer
GetHourOfTime = Hour(timeVal)
End Function
This `GetHourOfTime` function takes a time value as an argument and returns the hour part component. You could use this function in your VBA code or even as a worksheet function if you make it a public function in a module.