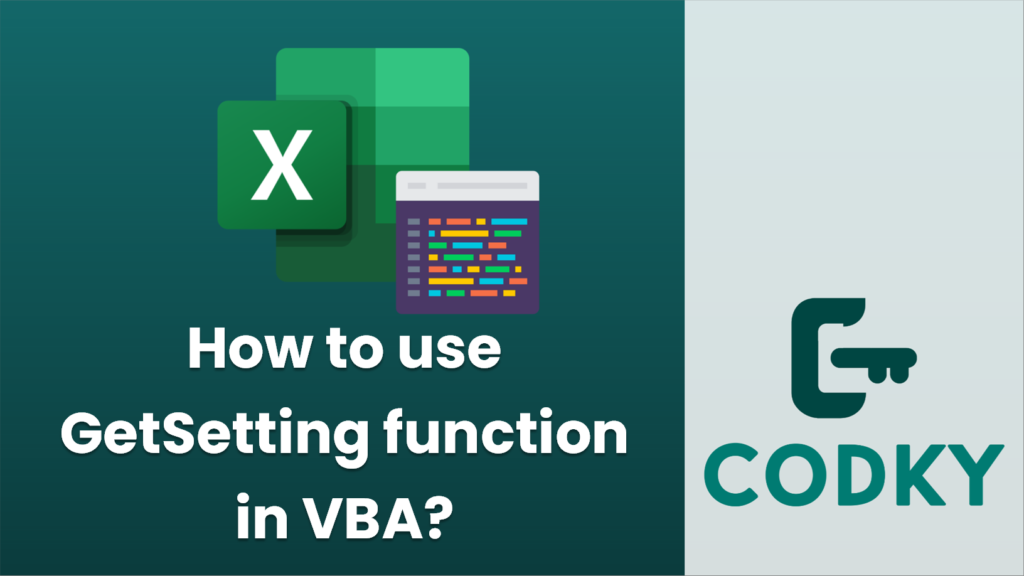
The `GetSetting` function in VBA allows you to retrieve a value from the Windows Registry. It’s useful for recalling user preferences or settings for an application you’ve written that are stored in the Registry. Note that this function is generally used to read settings written to the Registry by the `SaveSetting` function in VBA, rather than reading arbitrary Registry keys.
The syntax for the `GetSetting` function is as follows:
GetSetting(appname, section, key, [default])
Here’s what each of the parameters means:
- `appname` (String): The name of your application. This becomes a subkey under the `HKEY_CURRENT_USERSoftwareVB and VBA Program Settings` in the Registry where your settings are stored.
- `section` (String): The section under your application’s settings where the key is stored.
- `key` (String): The name of the key you want to retrieve the setting for.
- `default` (Optional Variant): The value to return if the key is not found. If omitted, it defaults to an empty string (“” for String values).
Example usage:
Sub RetrieveSettings()
' Define variables
Dim myAppName As String
Dim mySection As String
Dim myKey As String
Dim defaultValue As Variant
Dim settingValue As Variant
' Assign values to variables
myAppName = "MyVBAApp"
mySection = "Settings"
myKey = "WindowPosition"
defaultValue = "100,100" ' Default window position
' Retrieve the setting from the Registry
settingValue = GetSetting(myAppName, mySection, myKey, defaultValue)
' Display the setting value
MsgBox "The window position setting is: " & settingValue
End Sub
In this example, `GetSetting` will look for the key named “WindowPosition” under the section “Settings” for the application “MyVBAApp”. If it finds the key, it returns its value. If the key is not found, it returns the default value of “100,100”.
Important considerations when using `GetSetting`:
- Your VBA project needs to have permission to access the Windows Registry, which may be restricted in some environments.
- The `GetSetting` function is designed for light usage, such as storing user preferences for an application, rather than as a replacement for a proper database or file-based configuration system.
- Any settings saved with `SaveSetting` are typically stored per user, not system-wide, because they are written to `HKEY_CURRENT_USER`.
- The `GetSetting` function is not available in Mac versions of Excel.
- Be cautious when accessing the Windows Registry, as incorrect usage could potentially cause system stability issues.
If you need to interact with the Windows Registry more extensively or read system-wide settings, you will need to use Windows API calls instead of the VBA-specific `GetSetting` function.