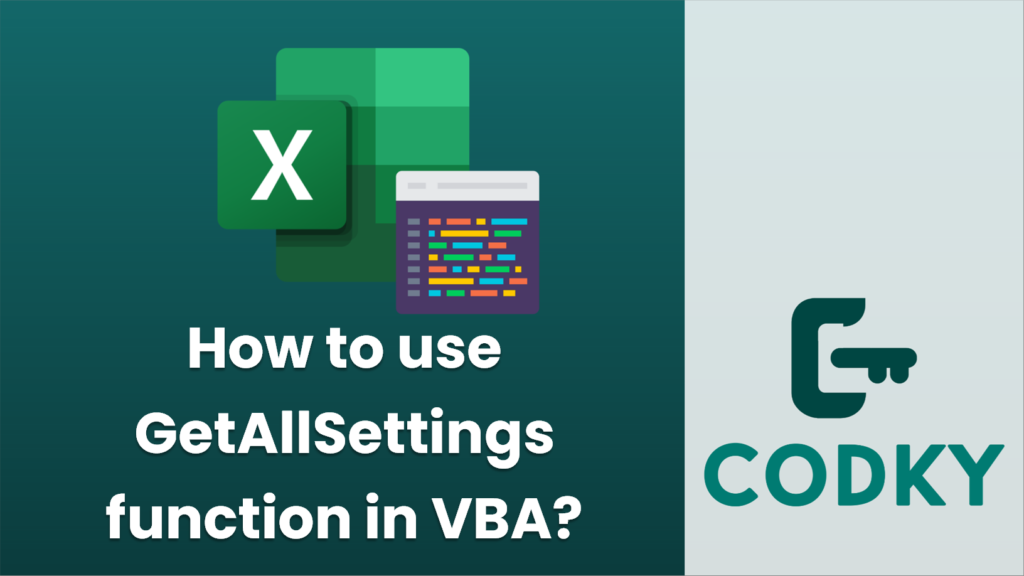
`GetAllSettings` is a function in Visual Basic for Applications (VBA) that retrieves all the setting names and their corresponding values for an application from the Windows registry. This function is particularly useful when you have previously used the `SaveSetting` function to store multiple settings and wish to retrieve them all at once.
Here’s how you can use `GetAllSettings` in VBA:
- Ensure that you have used the `SaveSetting` function beforehand to store some settings for your application.
- Use the `GetAllSettings` function in your VBA code by providing the application name and the section within the registry where the settings are stored.
Here’s an example of how to use the `GetAllSettings` function:
Sub RetrieveSettings()
Dim AppName As String
Dim SectionName As String
Dim settings As Variant
Dim i As Integer
' Specify the application name and section name exactly as you used them with SaveSetting
AppName = "MyApp"
SectionName = "Settings"
' Retrieve all the settings for the specified application and section
settings = GetAllSettings(AppName, SectionName)
' Check if any settings were retrieved
If Not IsEmpty(settings) Then
' Loop through the settings array and print them
For i = LBound(settings, 1) To UBound(settings, 1)
Debug.Print "Key: " & settings(i, 0) & " - Value: " & settings(i, 1)
Next i
Else
Debug.Print "No settings found for the specified application and section."
End If
End Sub
The function returns a two-dimensional array, with each row representing a setting. The first column (index 0) contains the setting name (the key), and the second column (index 1) contains the setting value.
Keep in mind the following:
- The `GetAllSettings` function works with the Windows registry. Due to user permissions and registry redirection on 64-bit systems, you might encounter limitations or differences in behavior based on the version of Windows and the Office application you’re using.
- Always ensure that your code gracefully handles the case where no settings are found (as shown in the example).
- In modern Windows systems and VBA practices, usage of the registry is generally discouraged in favor of other settings storage mechanisms, due to permissions and security concerns. Consider using alternative methods for storing settings, such as configuration files or databases, especially if you are developing new applications.