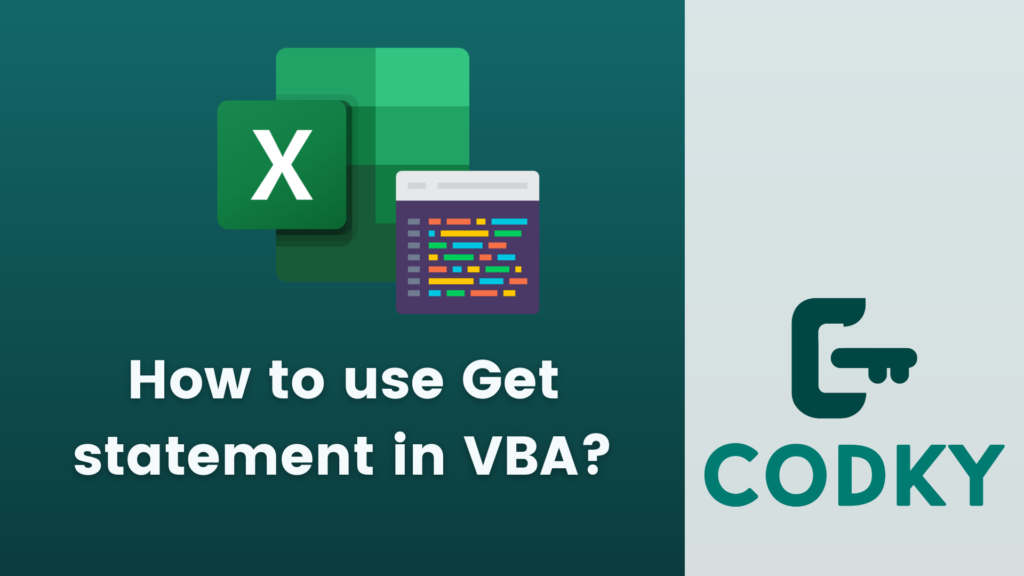
Syntax:
VBA
Get [#]fileNumber, [recordNumber], variable
- fileNumber: The file number used in the Open statement. It’s a unique number that identifies the open file.
- recordNumber: Optional. The record number at which the reading starts in the file. If omitted, the next record after the last Get or Put statement is read.
- variable: The name of the variable or array into which the data from the file will be read.
Using Get Statement:
- Open a File: First, you need to open a file using the Open statement.
- Read Data: Use the Get statement to read data from the file into a variable.
- Close the File: After completing the read operation, close the file using the Close statement.
Example:
Here’s a simple example of how to use the Get statement:VBA
In this example, a file named example.dat is opened for binary access. Data is read into the MyData variable, and then the file is closed. The MsgBox function is then used to display the data read from the file.
Dim MyData As String * 5 ' Define a fixed-length string.
Dim FileNum As Integer
FileNum = FreeFile() ' Get an available file number.
' Open a file for binary access.
Open "C:example.dat" For Binary As #FileNum
' Read data from the file into the variable.
Get #FileNum, , MyData
' Close the file.
Close #FileNum
' Display the data.
MsgBox MyData
Key Points:
- The Get statement is mainly used for binary file access, but it can also be used with Random and Input modes.
- Ensure the variable type matches the data type stored in the file to prevent errors or unexpected results.
- Remember to always close the file after completing the read operation.
- Error handling is recommended when dealing with file operations to manage exceptions such as file not found or access violations.
- It’s generally used in more traditional or legacy VBA applications, as more modern techniques for file handling might involve other methods or even different programming languages.