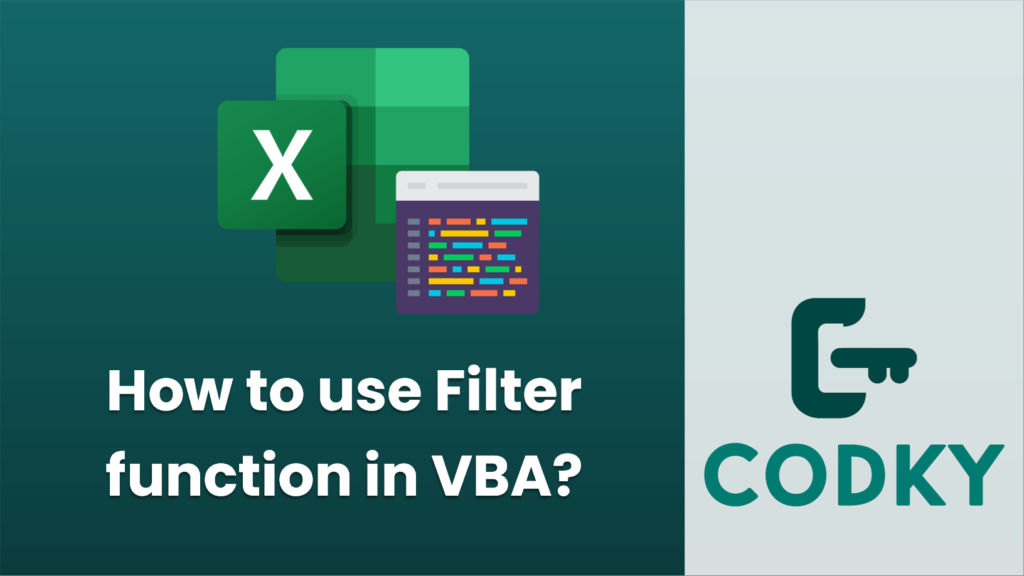
Basic syntax
VBA
Filter(SourceArray, Match, Include, [Compare])
Parameters
- SourceArray: The existing one-dimensional array of strings that you want to filter.
- Match: The substring you want to match in the array elements.
- Include: A Boolean value indicating whether to include (True) or exclude (False) the array elements that match the filter string.
- Compare: Optional. A numeric value specifying the type of comparison to use when comparing array elements with the filter string. 0 for a binary comparison (case-sensitive) or 1 for a textual comparison (case-insensitive).
Example Usage
Here’s a simple example showing how to use the Filter function in VBA:VB
After running this code, the Immediate Window would display the following output:
Sub FilterExample()
Dim arr() As String
Dim result() As String
' An array of strings
arr = Array("apple", "banana", "cherry", "date", "fig", "grape")
' Using the Filter function to find elements that contain "ap"
result = Filter(arr, "ap", True) ' Include elements that contain "ap"
' Print the results to the Immediate Window (Ctrl+G to view)
Dim i As Integer
For i = LBound(result) To UBound(result)
Debug.Print result(i)
Next i
End Sub
- apple
- grape