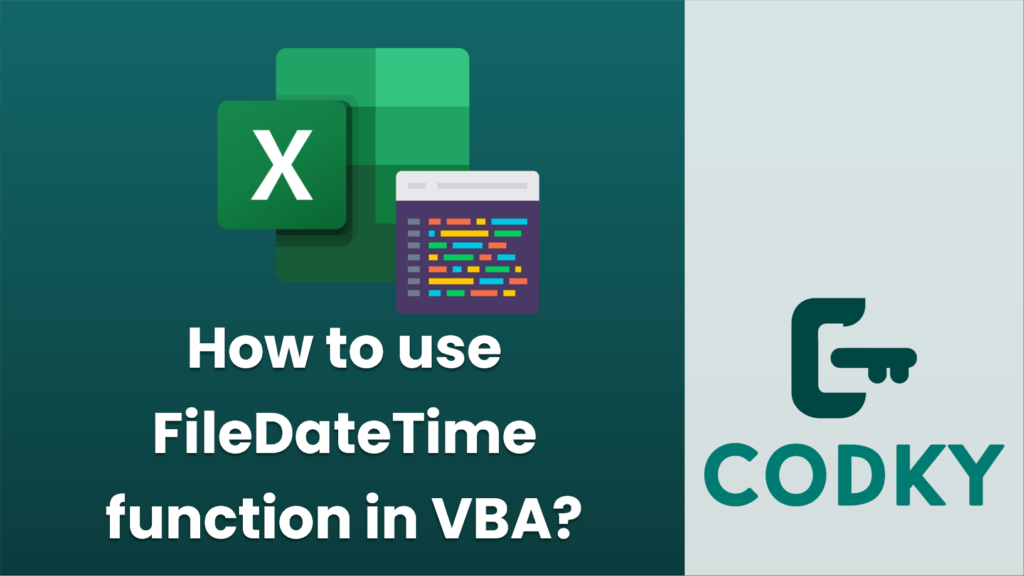
Contents
The FileDateTime function in VBA is used to return the date and time when a file was created or last modified. This function takes a single argument, which is the path to the file.
Basic Syntax:
VBA
FileDateTime(pathname)
pathname: A string expression that specifies a file. The pathname can include the directory or folder, and the drive.
Example
VBA
Sub ExampleFileDateTime()
Dim strFilePath As String
Dim dtFileCreated As Date
' Specify the path to the file
strFilePath = "C:\path_to_your_file\example.txt"
' Use FileDateTime to get the date and time of the file
dtFileCreated = FileDateTime(strFilePath)
' Display the date and time in a message box
MsgBox "The file was created or last modified on: " & dtFileCreated
End Sub
Note that the FileDateTime function will return an error if the file does not exist or the path is invalid. Therefore, it is good practice to check if the file exists before calling this function. You can use the Dir function to check if a file exists:
VBA
Sub ExampleFileDateTimeSafe()
Dim strFilePath As String
Dim dtFileCreated As Date
' Specify the path to the file
strFilePath = "C:\path_to_your_file\example.txt"
' Check if the file exists
If Len(Dir(strFilePath)) > 0 Then
' File exists, get the date and time
dtFileCreated = FileDateTime(strFilePath)
' Display the date and time in a message box
MsgBox "The file was created or last modified on: " & dtFileCreated
Else
' File does not exist
MsgBox "The specified file does not exist."
End If
End Sub
Remember to change “C:\path_to_your_file\example.txt” to the actual path of the file you want to check.