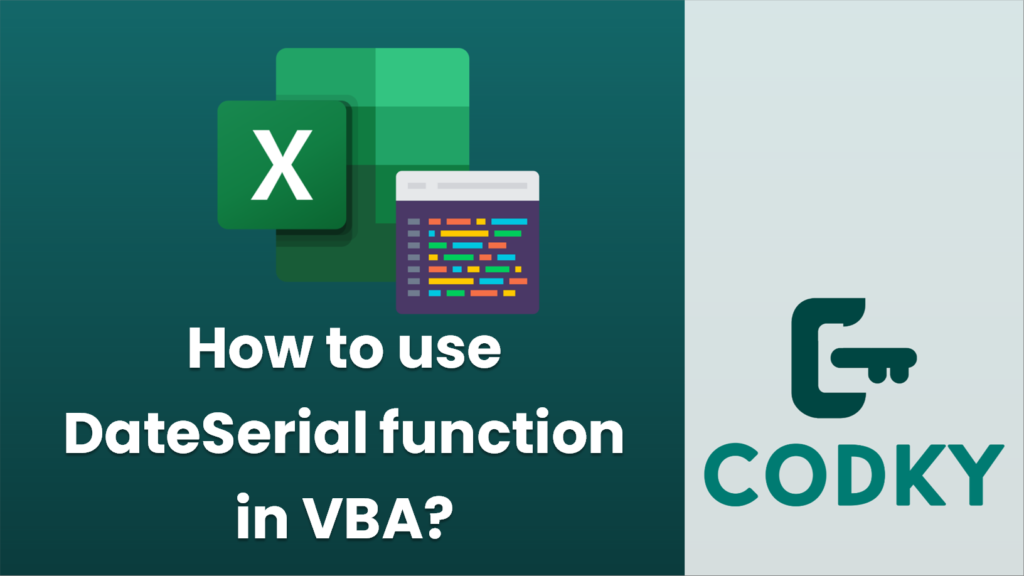
The DateSerial function in VBA (Visual Basic for Applications) is used to return a date value representing a specific year, month, and day. The syntax for the function is as follows:
DateSerial(year, month, day)
Where:
- year is the year part of the date. It can be a positive or negative integer.
- month is the month part of the date, between 1 (January) and 12 (December).
- day is the day part of the date. The day can be a number representing the day of the month.
If you specify out-of-range values for year, month, or day, DateSerial automatically adjusts them. For example, if you specify a month value of 13, DateSerial treats it as January of the following year.
Here’s an example of how you could use the DateSerial function in VBA:
Sub ExampleUseOfDateSerial()
Dim specificDate As Date
' Create a date representing December 31, 2023
specificDate = DateSerial(2023, 12, 31)
' Output the created date to the Immediate Window (press Ctrl + G to view)
Debug.Print "The specificDate is: " & specificDate
' Create a date using variables
Dim yearPart As Integer
Dim monthPart As Integer
Dim dayPart As Integer
yearPart = 2025
monthPart = 5
dayPart = 10
' Combine the parts into a date
specificDate = DateSerial(yearPart, monthPart, dayPart)
' Output the created date to Immediate Window
Debug.Print "The specificDate with variables is: " & specificDate
' Example with out-of-range values DateSerial will adjust them
Dim adjustedDate As Date
adjustedDate = DateSerial(2023, 13, 1) ' Represents January 1, 2024
Debug.Print "The adjustedDate is: " & adjustedDate
End Sub
In the above example, we’ve created a sub-procedure ExampleUseOfDateSerial which demonstrates using DateSerial with hard-coded values, with variables, and with out-of-range values.
Remember to execute this code from a VBA editor, which you can generally access in Excel by pressing ALT + F11 and inserting a module if you’re working with Excel VBA.