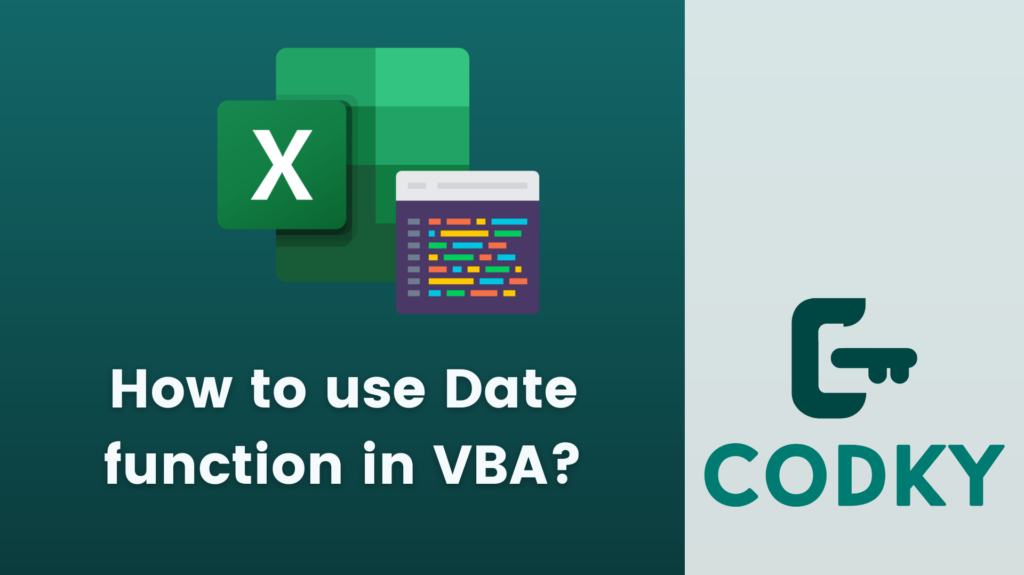
Basic Usage
To get the current date, simply use the Date function:VBA
This assigns the current system date to the variable currentDate.
Dim currentDate As Date
currentDate = Date
Displaying the Date
You can display the date using the MsgBox function or output it to a sheet in Excel:VBA
Or, if you are using it in Excel:
MsgBox "Today's date is " & Date
VBA
ActiveSheet.Range("A1").Value = Date
Formatting the Date
To format the date, you can use the Format function:VBA
This will format the date in the day/month/year format.
Dim formattedDate As String
formattedDate = Format(Date, "dd/mm/yyyy")
Using Date in Calculations
The Date function can be used in date calculations. For example, to find a date that is 10 days from now:VBA
Similarly, you can subtract days:
Dim futureDate As Date
futureDate = Date + 10
VBA
Dim pastDate As Date
pastDate = Date - 10
Comparing Dates
You can also use the Date function to compare dates:VBA
If Date > someOtherDate Then
MsgBox "Today's date is after someOtherDate."
End If
Using Date with Time
If you need the current date and time, use the Now function instead:VBA
Dim currentDateTime As Date
currentDateTime = Now
Getting Parts of a Date
To get specific parts of the date, like the year, month, or day, you can use Year, Month, and Day functions:VBA
Dim year As Integer
Dim month As Integer
Dim day As Integer
year = Year(Date)
month = Month(Date)
day = Day(Date)
Note
- The Date function always returns the current system date as set on the user’s computer.
- Remember that date formats might appear differently based on the system’s regional settings.