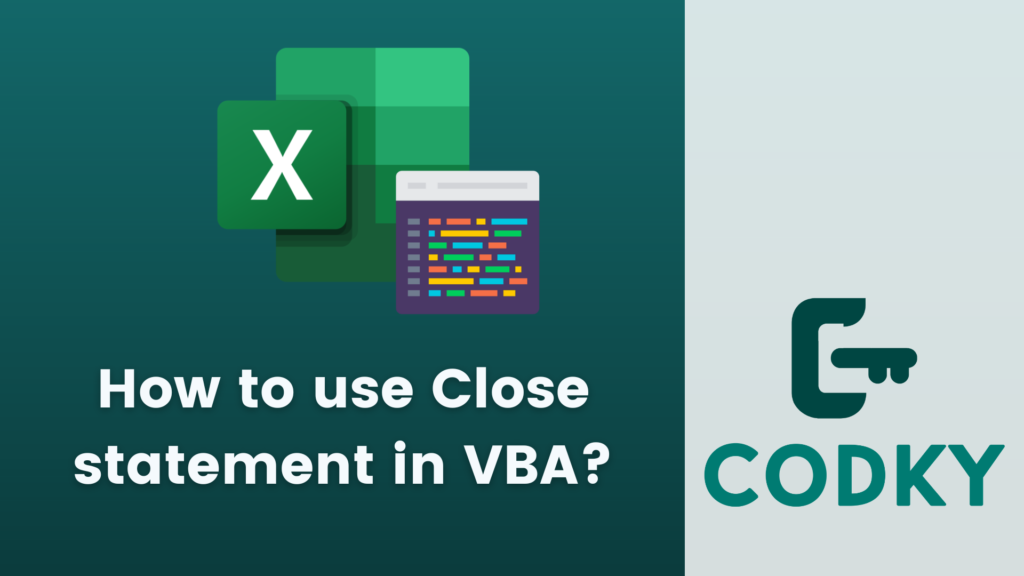
Contents
The Close statement in VBA (Visual Basic for Applications) is used to close files that have been opened in VBA with the Open statement. This is important for resource management, as it frees up the file to be used by other processes or applications and ensures that all the data is properly written and saved.
Here’s a basic overview of how to use the Close statement in VBA:
Open a File: Before you can close a file, you need to open it. This is done using the Open statement. For example:
Open "C:\MyFile.txt" For Output As #1
This line of code opens a file named “MyFile.txt” for output (writing to the file) and associates it with the file number 1.
Write or Read Data (optional): Depending on how you’ve opened the file (for input, output, or append), you can read from it, write to it, or append data to it.
Close the File: Once you are done with the file, you use the Close statement to close it. The syntax is straightforward:
Close #1
This line of code closes the file associated with file number 1.
If you have multiple files open, you can close them all at once by simply using Close without a file number:
Close
This will close all open files.
Here is an example of a complete VBA routine that opens a file, writes some data, and then closes the file:
Sub WriteToFile()
Dim filePath As String
filePath = "C:\MyFile.txt"
' Open the file for output
Open filePath For Output As #1
' Write some data to the file
Print #1, "Hello, world!"
' Close the file
Close #1
End Sub
In this subroutine, a text file is opened for output, a line of text is written to it, and then the file is closed. This is a basic pattern for file operations in VBA. Always remember to close files when you’re done with them to avoid locking issues and to ensure data integrity.