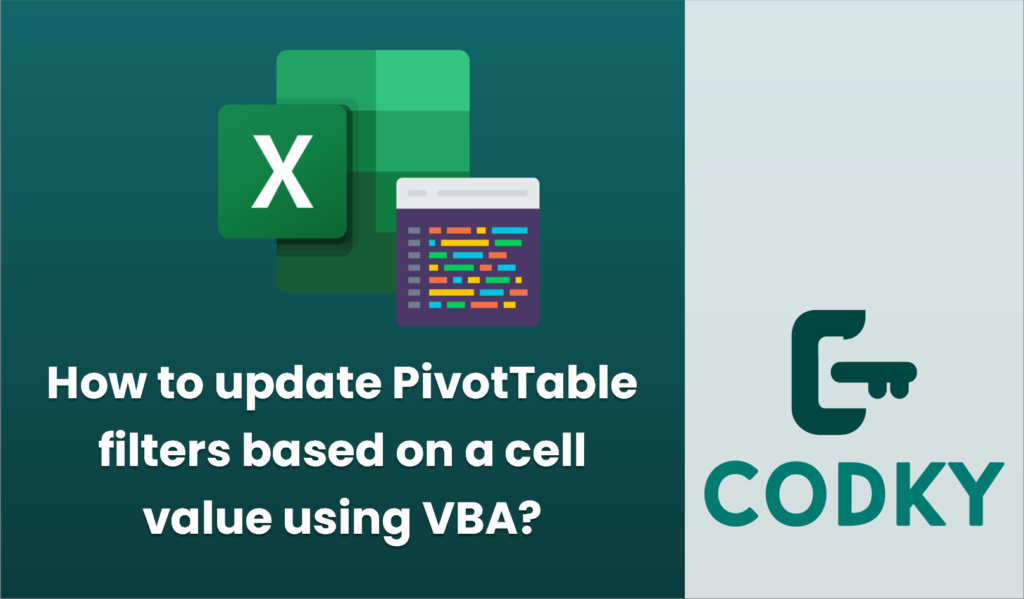
Updating PivotTable filters based on a cell value using VBA can be extremely useful for dynamic reporting and dashboards. Below is an example of how you can achieve this in Excel VBA:
Let’s assume you have:
- A PivotTable named “PivotTable1” on a worksheet named “Sheet1”.
- A filter field titled “Category”.
- A cell (e.g., B2) where the user inputs the desired filter value.
Here’s a step-by-step guide using VBA to update the PivotTable filter based on the cell value:
- Open the VBA Editor:
- Press `ALT + F11` to open the Visual Basic for Applications editor.
- Insert a Module:
- Click `Insert` > `Module` to create a new module.
- Write the VBA code:
Below is a sample VBA code that updates the PivotTable filter based on the value in cell B2.
Sub UpdatePivotFilter()
Dim ws As Worksheet
Dim pt As PivotTable
Dim filterValue As String
' Set worksheet and pivot table
Set ws = ThisWorkbook.Worksheets("Sheet1")
Set pt = ws.PivotTables("PivotTable1")
' Retrieve the filter value from cell B2
filterValue = ws.Range("B2").Value
' Ensure the PivotTable has a filter for the required field
On Error GoTo ErrorHandler
With pt.PivotFields("Category")
' Clear any previous filter
.ClearAllFilters
' Apply filter based on cell B2 value
.PivotItems(filterValue).Visible = True
End With
Exit Sub
ErrorHandler:
MsgBox "An error occurred. Please ensure the filter value is valid.", vbExclamation
End Sub
- Execute the Macro:
- You can run this macro by pressing `F5` in the VBA editor or by assigning it to a button in Excel.
Notes:
- Error Handling: The included error handler displays a message if the provided filter value does not exist in the PivotTable field.
- Multiple Filters: If you have multiple fields to filter, replicate the `With…End With` block for each field and adjust accordingly.
- Dynamic Inputs: This solution assumes a specific layout and known field names. Adjust the references for actual scenarios.
- Valid Data: Ensure the data in the filter field (e.g., “Category”) matches the possible entries in the PivotTable for the code to work correctly.
You can further customize this script to meet specific needs, such as handling multiple field types (numbers, dates) or combining multiple filters.