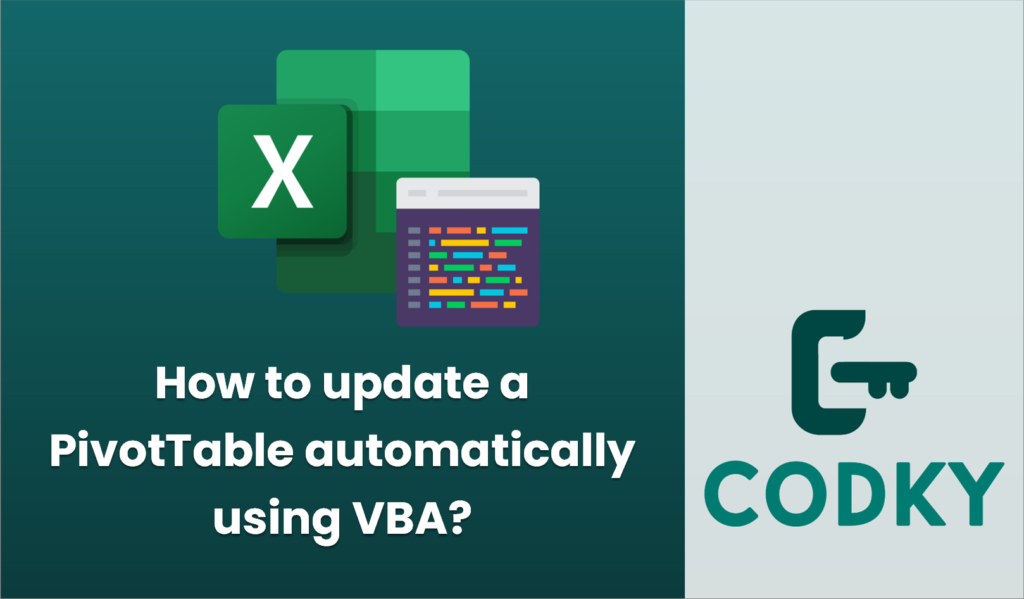
To update a PivotTable automatically using VBA, you can write a macro that refreshes the PivotTable whenever necessary, such as when the workbook opens, a particular worksheet is activated, or specific data changes. Here’s a general guide and example using VBA to achieve this:
Steps:
Sub RefreshPivotTables()
Dim ws As Worksheet
Dim pt As PivotTable
' Loop through each worksheet in the active workbook
For Each ws In ThisWorkbook.Worksheets
' Loop through each PivotTable in the worksheet
For Each pt In ws.PivotTables
pt.PivotCache.Refresh
Next pt
Next ws
End Sub
Private Sub Workbook_Open()
RefreshPivotTables
End Sub
Private Sub Worksheet_Activate()
RefreshPivotTables
End Sub
- Open the VBA Editor:
- Press `ALT + F11` to open the VBA editor in Excel.
- Insert a New Module:
- In the VBA editor, right-click on any of your existing project folders and select `Insert > Module`.
- Write the VBA Code:
- You can use the following VBA code as a starting point. This code will refresh all PivotTables in the active worksheet or a specific worksheet:
- Attach the Macro to an Event:
- You can call `RefreshPivotTables` macro from various events. For instance, to refresh PivotTables whenever the workbook opens, put this code in the `Workbook_Open` event:
- To attach this to a specific worksheet activate event, place it in the `Worksheet_Activate` event:
- Save Your Workbook:
- Save your workbook as a macro-enabled workbook (`.xlsm`) to ensure your VBA code is saved.
- Run the Macro:
- If you want to run the macro manually, you can press `ALT + F8`, then select `RefreshPivotTables` and click `Run`.
Additional Notes:
- Specific Worksheet: If you want to refresh PivotTables only on a specific worksheet, replace `For Each ws In ThisWorkbook.Worksheets` with something like `Set ws = ThisWorkbook.Sheets(“Sheet1”)`.
- Protecting Your Code: If necessary, protect your VBA project with a password to prevent unauthorized changes to your code.
By following these steps, you can ensure that your PivotTables are updated automatically according to your specified criteria.