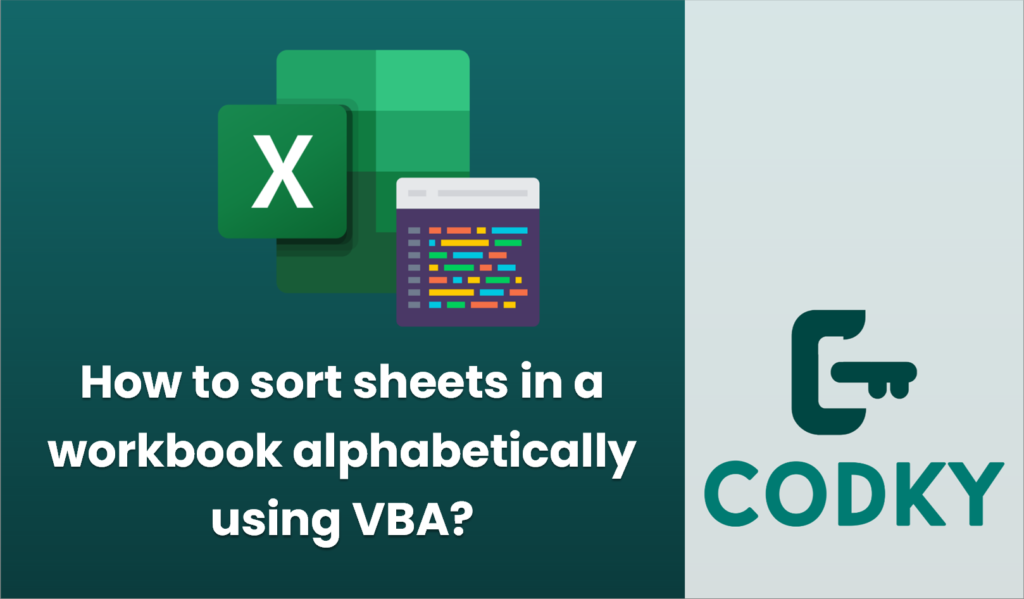
Sorting sheets in a workbook alphabetically using VBA can be achieved with a simple macro. Below is a step-by-step guide and the corresponding VBA code to accomplish this:
Step-by-Step Guide:
Sub SortSheetsAlphabetically()
Dim i As Integer, j As Integer
Dim iAnswer As VbMsgBoxResult
' Prompt the user for confirmation
iAnswer = MsgBox("Do you want to sort the sheets alphabetically?", vbYesNo + vbQuestion, "Sort Sheets")
If iAnswer = vbNo Then Exit Sub
' Loop through all sheets and sort them alphabetically
For i = 1 To ThisWorkbook.Sheets.Count - 1
For j = i + 1 To ThisWorkbook.Sheets.Count
If UCase(ThisWorkbook.Sheets(j).Name) < UCase(ThisWorkbook.Sheets(i).Name) Then
ThisWorkbook.Sheets(j).Move Before:=ThisWorkbook.Sheets(i)
End If
Next j
Next i
MsgBox "Sheets have been sorted alphabetically.", vbInformation, "Sort Complete"
End Sub
- Open Excel: Open the Excel workbook where you want to sort the sheets.
- Open the VBA Editor: Press `ALT` + `F11` to open the Visual Basic for Applications (VBA) editor.
- Insert a Module: In the VBA editor, go to `Insert` > `Module` to create a new module.
- Copy the Code: Paste the following VBA code into the module:
- Run the Macro: Close the VBA editor and go back to Excel. Press `ALT` + `F8` to open the “Macro” dialog box, select `SortSheetsAlphabetically`, and click “Run”.
Explanation:
- Prompt: The macro starts by asking the user for confirmation to sort the sheets.
- Sorting Logic: It uses a simple bubble sort algorithm to compare the names of the sheets and moves a sheet before another if its name is alphabetically earlier.
- Case Insensitivity: It converts names to uppercase using `UCase()` for a case-insensitive comparison.
- Notification: After sorting, a message box informs the user that the operation is complete.
Ensure that your workbook is saved before running any macros, as the sort operation cannot be undone using the regular Excel undo command. Also, if your workbook contains any hidden sheets, they will remain hidden and included in the sort operation.