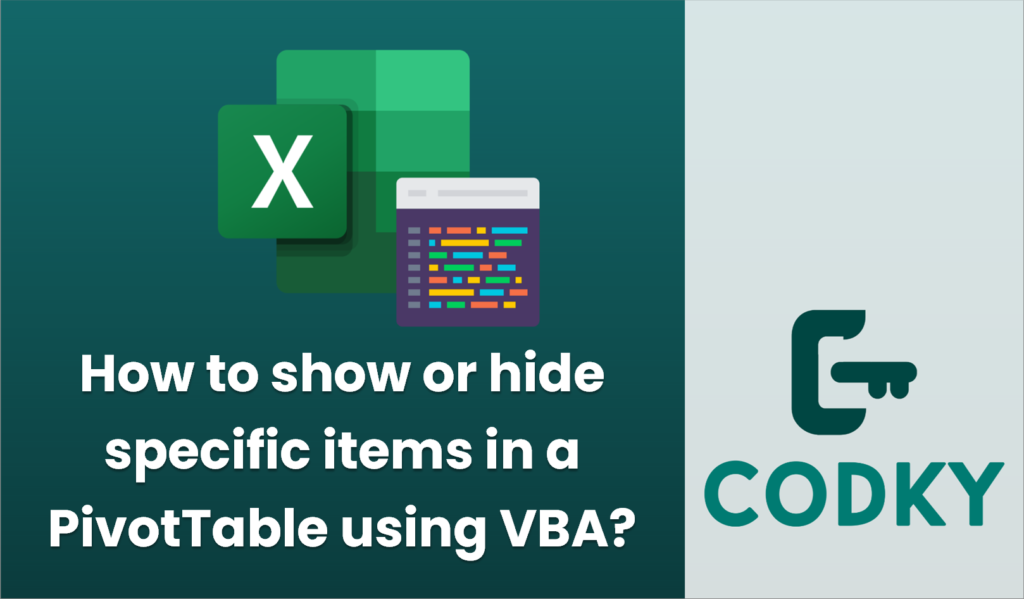
To show or hide specific items in a PivotTable using VBA, you need to interact with the `PivotItems` collection of the particular `PivotField` you want to modify. Here’s a step-by-step guide on how to do this:
Steps to Show/Hide Items in a PivotTable using VBA:
- Open the Visual Basic for Applications Editor:
- Press `ALT + F11` to open the VBA editor in Excel.
- Insert a New Module:
- Right-click on any of the objects for your workbook in the Project Explorer.
- Select `Insert > Module` to create a new module.
- Write the VBA Macro:
Here’s an example code to show or hide specific items in a PivotTable:
Sub ShowHidePivotItems()
Dim ws As Worksheet
Dim pt As PivotTable
Dim pf As PivotField
Dim pi As PivotItem
' Set the worksheet and pivot table
Set ws = ThisWorkbook.Sheets("YourSheetName") ' Change to your sheet name
Set pt = ws.PivotTables("YourPivotTableName") ' Change to your PivotTable name
' Set the pivot field you want to modify
Set pf = pt.PivotFields("YourFieldName") ' Change to your field name
' Loop through all pivot items in the field
For Each pi In pf.PivotItems
' Check the item value and hide/show
Select Case pi.Name
Case "Item1", "Item2" ' Specify items you want to work with
pi.Visible = True ' Set to True to show or False to hide
Case Else
pi.Visible = False ' Show or hide all other items
End Select
Next pi
End Sub
Important Points:
- Run the Macro:
- Close the VBA Editor and go back to Excel.
- Press `ALT + F8`, select `ShowHidePivotItems`, and click `Run` to execute the macro.
Notes:
- Ensure that the PivotTable is up-to-date and the field names are correct to avoid runtime errors.
- This macro can also be modified to handle multiple fields or complex conditions based on your requirements.
By running this VBA macro, you can easily control the visibility of specific items in your PivotTable dynamically.