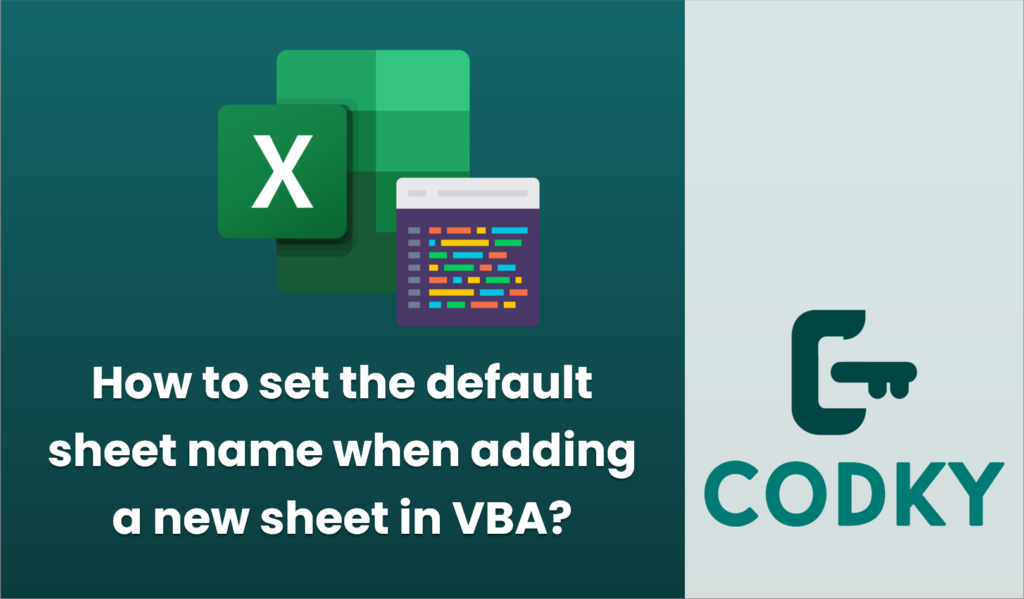
In VBA, when you add a new sheet to a workbook using the `Worksheets.Add` method, Excel automatically assigns a default name like “Sheet2” or “Sheet3.” However, you can set a specific name for the sheet right after adding it. Here’s how you can do it:
Sub AddSheetWithName()
Dim ws As Worksheet
' Add a new sheet
Set ws = Worksheets.Add
' Set the default name for the new sheet
ws.Name = "YourDesiredSheetName"
End Sub
Make sure to replace `”YourDesiredSheetName”` with the name you want to assign to the new sheet. Keep in mind that sheet names must be unique within a workbook and cannot exceed 31 characters. Additionally, they cannot contain any of the following characters: / ? * [ ].
If you have specific logic for determining the sheet name (e.g., incrementing numbers if a name already exists), you might need to add additional code to handle those cases. Here’s an example that ensures the sheet name is unique:
Sub AddSheetWithUniqueName()
Dim ws As Worksheet
Dim sheetName As String
Dim i As Integer
Dim nameExists As Boolean
i = 1
nameExists = True
' Loop until a unique name is found
Do While nameExists
sheetName = "SheetName" & i
nameExists = False
For Each ws In Worksheets
If ws.Name = sheetName Then
nameExists = True
Exit For
End If
Next ws
i = i + 1
Loop
' Add a new sheet with the unique name
Set ws = Worksheets.Add
ws.Name = sheetName
End Sub
This code tries to name the new sheet “SheetName1”, “SheetName2”, etc., until it finds a name that is not already used by another sheet in the workbook.