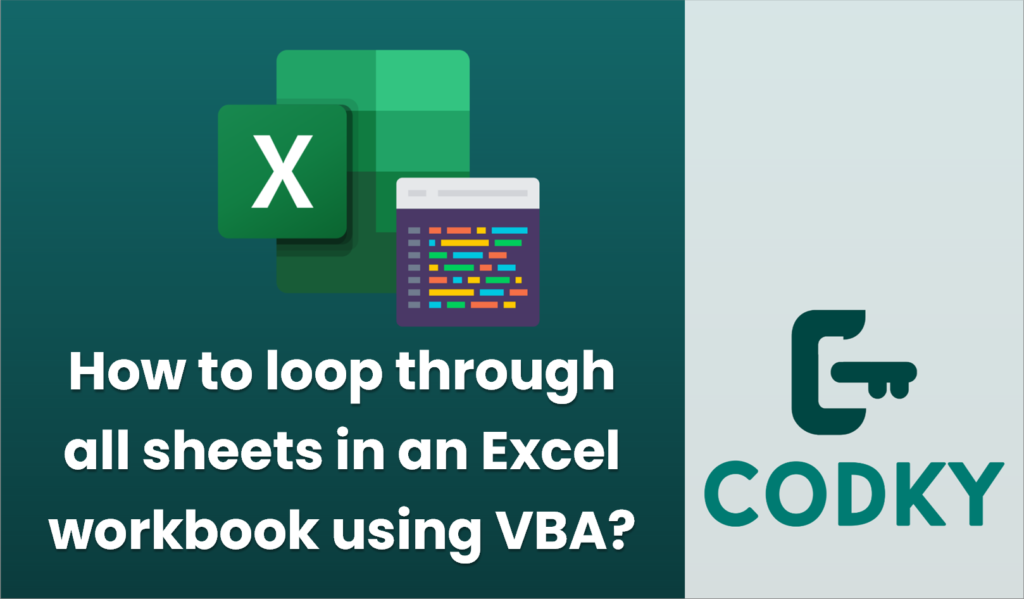
To loop through all sheets in an Excel workbook using VBA (Visual Basic for Applications), you can use the `Worksheets` or `Sheets` collection in a loop. This allows you to perform operations on each sheet within the workbook. Below is a basic example that demonstrates how you can do this:
Sub LoopThroughSheets()
Dim ws As Worksheet
' Loop through each worksheet in the active workbook
For Each ws In ThisWorkbook.Worksheets
' You can perform actions on each worksheet here
' For example, display the worksheet name in a message box
MsgBox "Sheet Name: " & ws.Name
Next ws
End Sub
Explanation:
- Dim ws As Worksheet: This creates a variable named `ws` to represent each worksheet.
- For Each ws In ThisWorkbook.Worksheets: This starts a loop through each worksheet in the workbook that contains the code (`ThisWorkbook`).
- MsgBox “Sheet Name: ” & ws.Name: Inside the loop, you can perform any action you need on the worksheet. Here, it shows a message box with the name of each worksheet.
- Next ws: This finishes the loop and moves to the next worksheet.
Additional Notes:
Dim sh As Object
For Each sh In ThisWorkbook.Sheets
MsgBox "Sheet/Chart Name: " & sh.Name
Next sh
- If you want to include Chart Sheets in addition to regular Worksheets, you can loop through the `Sheets` collection instead of `Worksheets`:
- Replace `ThisWorkbook` with `ActiveWorkbook` if you want to refer to the workbook that’s currently open and active rather than the one containing the code.
- You can customize the operations inside the loop per your specific needs, such as modifying cell values, formatting sheets, or copying data.